Computed Properties vs Watch in Vue JS
In this video you will learn what is computed and watch things in Vue and how you can use them for your own benefit.
In previous video we implemented API call for getting our users name. Now it's time to talk about 2 super important things which makes coding with Vue so much easier.
First is computed properties. What is this? So you can create properties in Vue component based on other properties. Let's look on our UsersList component. We know that we are getting the array of users in our component. But for example we need to create a property which has all names of this users inside the component. How can we do that? The first approach will be to write the code inside mounted. So when component is mounted on initialize we are creating array of names. This can be fine on the first glance but if our array of users changes then it won't work. And actually our array of users changes because at the beginning we are passing in our component users as an empty array and then after we fetched them as an array of objects.
Exactly for this purpose we have computed properties. Let's create it now.
computed: {
userNames() {
return this.users.map(user => user.name)
}
}
So computed is a special property inside our object. The name of the function (in our case userNames) is our computed property which will be available just like any normal property inside this. Here we simply can return whatever we want. It can be just a string for example or any properties that you want to use to create a computed property. The coolest thing here is that every time when any of your dependencies change this computed property will change. So in our case when users array changes our userNames property automatically changes.
As you can see in browser we have in template access to the array of user names. Once again I can't stress it enough. Computed properties is one of the things that make writing code in Vue declarative. Because we define not what will happen like "When users array changes do this and that" but we declaratively define how properties are calculated and then simply use them. It simplifies the code and debugging enormously.
So I think it's clear with computed properties. Now let's talk about watch property. This is the same like computed but is completely the opposite. Let's add here watch section.
watch: {
users() {
this.userIds = this.users.map((user) => user.name);
},
},
So here we specify function with name of the property that we want to watch, So actually this is a callback when users property will be changes. Then inside we are doing any code that we need. Here we assigned all userIds to this.userIds. And as we don't have this property we need to define it inside data.
data() {
return {
newUserName: "",
userIds: [],
};
},
As you can see in browser we have the array of ids available. So now you might ask what is the difference and what you need to use? So as you see computed is a pure function which just calculates something and return. So it's a declarative approach to write code. Watch on the other hand is imperative way because we are writing what should happen by hands.
Actually 90% of the time I recommend you to write only computed properties. This will make your code safe and easy to understand. You should never use watch to just calculate some property based on other property. The need of the watch is when you want to retrigger something. For example you have a pagination and user clicks on other page. In this case your page number will change and you want to call API request. This is a correct case for watch. You want to watch a property and make API call.
So watch and computed are similar but completely different. Computed is pure and declarative and watch is completely imperative. If you can solve something with computed and without watch just do that. It will help you later.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done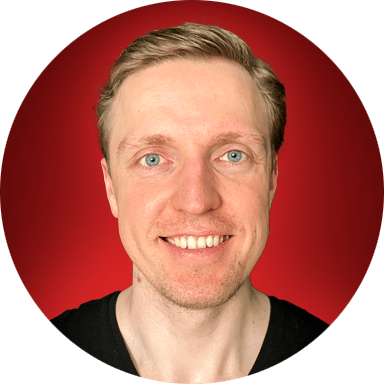