Create a Rest API With Json Server
A lot of times you want to do some frontend project or test something and you normally need an API for this. But it takes a lot of time and knowledge to create a real API. This is why in this video I want to show you the json-server npm package which helps you to create a completely real API in a matter of minutes.
So let's jump right into it.
So first of all make sure that you want this video until the end because I will share my bonus tip. The way how I'm building authentication using an extension for json-server.
So the first question that you might ask "Why don't we you thing or that service that allows us to create an own API?". First of all I like to keep things simple and avoid dependencies (especially external once) if possible because you never know when your dependency will stop working. Also I want to be in a full control of things that I'm running.
Why brings us to json-server package. As it's a node package you need to have some node version installed on your machine. If you don't know if you have node or not you can simply call
node -v
Now we need to install json-server globally because we will use it as a CLI tool.
npm install -g json-server
Now the main idea of json-server is that we build our database as a simple JSON in the file in special format. After that json-server can read and update this file (like a real database) and also generate API out of the box for us.
So here is my almost empty folder where I want to have an API. For this we simply need to create db.json file where the keys are entities that we want to create.
Let's make some posts.
{
"posts": [
{ "id": 1, "title": "json-server" },
{ "id": 2, "title": "node js" }
]
}
Our basic database with some data is ready and we can start our API now. We this we need to write in console.
json-server --watch db.json
As you can see we a getting a message that our API is already running. Also we can see all links to the entities that we created. If we jump to
http://localhost:3000/posts
We can see the list of posts.
What makes json-server really awesome is that we can make API calls like with real API. So it's not just get for the list of posts. We get call get by ID, but also make PUT, POST, PATCH requests which will automatically change the db.json file.
Also as you can see from documentation we can use filter, sorting and pagination for all our data out of the box. So if we write in url
http://localhost:3000/posts?title=json-server
Our data will be automatically filtered.
There is one more important thing to rememer. Normally you run your frontend for example React with create-react-app and you want to run parallel to it json-server. The problem here is that they both want to start on 3000 port. To avoid port collisions we can specify other port for json-server directly in CLI.
json-server --watch db.json --port 3004
Now let's check how it is working in real life. If you run some web server of React, Angular or Vue you can just use json-server as it is. Because both servers will be run in localhost and you can simply make requests between them.
Now on my machine I just have an index.html file and no webserver. So I can't just make calls to localhost and I need first to run somehow my folder on localhost also as json-server.
For this I will use another awesome package which is called serve. You can also install it will node. It allow you to do exactly that. Serve static files with a web server.
npm install -g serve
serve
When we write serve inside a folder with index.html file it starts a webserver with this html file. So how we can jump in
http://localhost:5000/
And we see our empty web page.
Now let's make a request for getting our posts from API.
<div id="posts"></div>
<script>
fetch('http://localhost:3000/posts').then(response => response.json()).then(posts => {
console.log(posts)
let nodes = posts.map(post => {
let el = document.createElement('div');
el.textContent = post.title;
return el;
});
document.getElementById('posts').append(...nodes)
});
</script>
As you can see we successfully made a request from our frontend to our API. And you can use this approach with any framework.
Now here is a bonus. Very often you also want to have an authentication for your frontend. And building it for real is time consuming. Json-server on it's own doesn't have anything about authentication but it is good archtectured and allows such things as modules. So you can write additional modules in a certain way and add it to json-server. And my favourite module is json-server-auth. It's a extension for json-server which allows you to build authentication and authorization flows on a certain levels. Of course it's not 100% as real authentication but it allows you to test a lot of functionality.
So first of all we need to install json-server-auth package.
npm install json-server-auth
But there is one problem that I had at this step. We install json-server-auth locally and it wants also to have json-server locally. So let's install json-server locally also.
npm install json-server
Now we can start json-server with json-server-auth extension.
json-server --watch db.json -m ./node_modules/json-server-auth
This gives us 3 new routes out of the box /register, /signup, /users. So we can register a user now and it's password will be correctly encrypted and stored in our db.json. For registration only email and password are required but we can throw inside any fields that we want additionally and they will be saved automatically. The response will give us an access token that we can store on frontend and attach to headers in order to tell our API that we are logged in.
The idea is that all urls are guarded out of the box and we can use different routes in order to test different access. For example
http://localhost:3000/640/posts
Means that only logged in user can access this route.
Let's try to register a user to see how it works.
document.getElementById('register').addEventListener('click', () => {
console.log('register')
fetch('http://localhost:3000/register', {
method: 'POST',
body: JSON.stringify({
email: 'monster@gmail.com',
password: '12345678'
}),
headers: {
'Content-Type': 'application/json'
},
}).then(response => response.json()).then(user => {
console.log('registered', user)
})
})
So here we registered a user. As you can see we need to create users property in db.json and json-serve-auth saved all data correctly and gives us accessToken back.
As you can see with json-server it's really easy to build a fake API server even with such complex thing as authorization.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done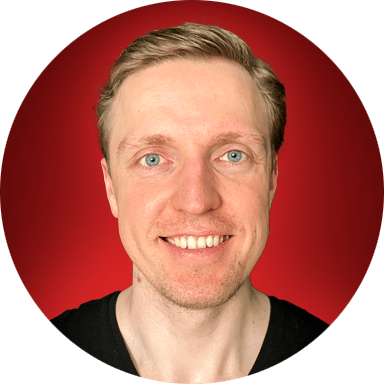