How to Animate Page Loading With CSS
In this video you will learn how to create a skeleton loading animation for you pages using plain css. It's a nice approach to show user that something is loading and it gives a feeling that your website is faster.
Let's jump right into it.
So the first question how we can do it? Actually it's not possible to build it like a fully reusable element that we can use on any website because the dimentions of elements and the skeleton can be different. But what we can for sure reuse is this gray elements with animation.
Let's create a single image to test.
<div class="image skeleton-box"></div>
.image {
width: 100px;
height: 80px;
}
.skeleton-box {
display: inline-block;
background-color: #DDDBDD;
}
Actually even rendering a bunch of gray element already can show the skeleton even without animation because normally we will see it for a second of so. But we want of course to make it fancy. We want this sliding animation from left to the right. Actually this is just an element with gradient which slides from left to the right so we can use after in order to render it inside skeleton-box.
.skeleton-box {
display: inline-block;
position: relative;
background-color: #DDDBDD;
}
.skeleton-box:after {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
background: red;
content: '';
}
As you can see red block takes the full size of our block. Now we need a keyframe animation to make this block moving.
.skeleton-box:after {
...
animation: slide 5s infinite;
}
@keyframes slide {
0% {
transform: translateX(-100%);
}
100% {
transform: translateX(100%);
}
}
As you can see it slides nicely because we moved it a bit to the left and to the right. Now we need to add overflow hidden to hide an element outside of our block.
.skeleton-box {
...
overflow: hidden;
}
This is exactly the animation that we want to achieve. Now let's pick a gradient to make it pretty.
.skeleton-box:after {
background: linear-gradient(to right, transparent 0%, #E8E8E8 50%, transparent 100%);
}
So now it's not super visible but gives the feeling of movement. This is exactly the class that we can reuse now for all our elements.
Now let's add some markup which is specific for the part of the page that we want to do. For example let's say that we are loading feed.
<div class="feed">
<div class="blog-post">
<div class="image skeleton-box"></div>
<div class="body">
<div class="skeleton-box headline"></div>
<div class="skeleton-box" style="width: 80%;"></div>
<div class="skeleton-box" style="width: 90%;"></div>
<div class="skeleton-box" style="width: 83%;"></div>
<div class="skeleton-box" style="width: 80%;"></div>
</div>
</div>
</div>
.feed {
max-width: 700px;
}
.blog-post {
display: flex;
margin-bottom: 30px;
}
.image {
width: 100px;
height: 80px;
}
.body {
margin-left: 20px;
width: 100%;
}
.headline {
width: 55%;
margin-bottom: 20px;
}
So here we used different width because then it gives a feeling that it's a real text of different length and not just gray blocks.
.skeleton-box {
...
height: 16px;
}
The only important part that we changed is that we added initial height for skeleton-box. It gives us a possibility to create elements and just get height of the single line out of the box.
And this is the most correct and easy way to implement skeleton loading animation for your pages. Of course if you are using some frontend framework like React it makes sense to hide all markup and css inside a single component and reuse it with just a single line.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done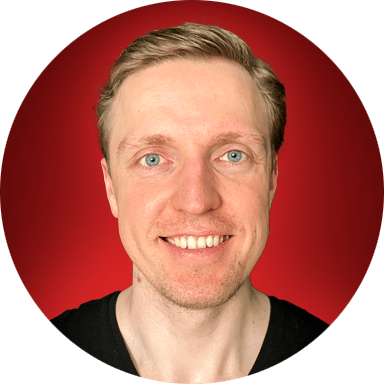