How to Use YouTube API in Node - Full Tutorial
In this video you will learn how to use youtube API inside NodeJS. So let's jump right into it.
So what we want to implement at all? We want to create an API route in our node application where we can provide a search string like in youtube seaarch box and get some results back. For example list of titles of videos that we searched for.
Actually it's possible to use youtube API only with key for your google account. It is done to avoid too many unauthorized requests. So our first step is to to API key so we can make requests to youtube. First we need to open google cloud console and create new project. If you don't have a google account you should register it first because without it you can't login to google cloud.
Now here we need to create new project and activate an API that we want to use. Let's search for youtube API and as you can see we have V3 now which is the newest.
Now let's jump to credentials tab and create a new API key. This is exactly what we need to make API requests.
The next step is to generate our node application. So here I have an empty folder so first let's create our package.json
npm init
Now let's install express. Why it? Because it's the most popular and small framework for Node from which we want routes and easy way to parse query parameters.
npm install express
Now let's create an express application and start it.
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello World!");
});
app.listen(port, () => {
console.log(`App listening at http://localhost:${port}`);
});
To start an application we write in console
node server.js
Now as you can see in browser our request is working.
Our next step is to create a new url where we can provide some search string and later get data from youtube API.
app.get("/search", async (req, res, next) => {
const searchQuery = req.query.search_query;
res.send(searchQuery);
});
As you can see our search url is working and because we used express we get out of the box possibility to read query parameters.
Now it's time to use API of youtube. As we want to get the list of videos like in search of youtube here is the request that we need for this.
https://www.googleapis.com/youtube/v3/search?key=apiKey&type=video&part=snippet&q=foo
So here we see v3 api and we provide several things. The most important for us is key. This is our API key that we already have. If we don't provide it we will get 403 error. The next part is q parameter. This is our search query like we provide in youtube search input. We also want to include part=snippet to get additional fields in the response like title, description, tags and channel id. We also want to filter our data by video type because there are much more like channels, playlists and much more.
Now let's use this use and fetch data from it. By default in NodeJS we don't have anything to fetch data from API. I highly recommend axios library which is the easiest way to work with API in browser and in NodeJS.
So let's install it first
npm install axios
And add fetch now. I will use async await notation to make code easier to read and support.
const axios = require("axios");
const apiKey = "AIzaSyDh4lSGdVGOnOg9oEvLTeLeCvl1eH5UMb0";
const apiUrl = "https://www.googleapis.com/youtube/v3";
app.get("/search", async (req, res, next) => {
try {
const searchQuery = req.query.search_query;
const url = `${apiUrl}/search?key=${apiKey}&type=video&part=snippet&q=${searchQuery}`;
const response = await axios.get(url);
const titles = response.data.items.map((item) => item.snippet.title);
res.send(titles);
} catch (err) {
next(err);
}
});
So here I stored apiKey and apiUrl in additional properties so we can reuse them in other requests later. we also prepare the correct url with params and call axios.get with await. Inside response we loop through all items to get titles of each element.
We also wrapped our code in try catch block so we can react on errors. The easiest way to handle errors is just to propagate it to Exress framework by using next.
As you can see in browser our code is working and we get our list of video titles in API.
And in this point you might ask why we create an API in NodeJS at all if we probably can use youtube API directly in client. For example in React application. And actually it is possible but it always makes sense to create your own API if you plan to use public API. First of all it's safe and you can store API keys there. In client side it's not safe. Secondly you can add any logic or normalize data inside oun API and not just throw lots of data that you don't need in your client.
Now actually there is an easier way to work with youtube API. There is an official package to work with it. But you must understand that this is just wrapper around fetch requests nothing more. So all code that we wrote already is just hidden inside a package. So let's try this out. For this we need to install googleapis package first.
npm install googleapis
Now we must create a youtube install and provide our key there.
const { google } = require("googleapis");
const youtube = google.youtube({
version: "v3",
auth: apiKey,
});
Now let's create a new page which will do exactly the same like in our first example but with this library.
app.get("/search-with-googleapis", async (req, res, next) => {
try {
const searchQuery = req.query.search_query;
const response = await youtube.search.list({
part: "snippet",
q: searchQuery,
});
const titles = response.data.items.map((item) => item.snippet.title);
res.send(titles);
} catch (err) {
next(err);
}
});
As you can see code works exactly the same but the only difference is that now our API call is hidden inside a library and we just call youtube.search.list where we provide exactly the same options.
Also I want to mention that using library is probably a better idea because if the new API version comes out it might be that all wrappers stay the same and just change API requests and logic inside. Which means maybe you only need to update an API version in config and nothing more. But if you use API directly probably you would need to change more code.
So now you know how to work with youtube API directly just by working with API url and through the library which simplifies it.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done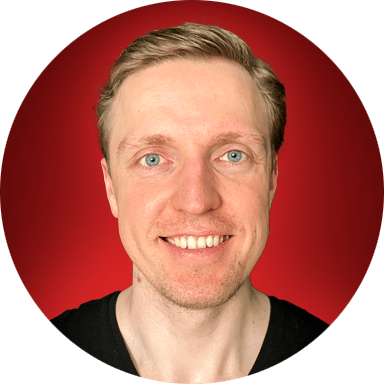