Making HTTP Requests in Vue
In this video you will learn how you can do API requests in Vue.
In all previous videos we worked only with data which where inside our Vue application. In the real project we fetch almost all data from the backend via API. Vue allows us make make API calls in an easy way. But here is the problem. We don't have any API to make our call and of course I don't want to make it too complex with implementing real backend with API.
But there is a nice solution for building Fake API. It's an npm package which is called json-server. I used it a lot for simple backends without complex logic.
Let's install it now.
npm install -g json-server
Now all that we need to do is create db.json file. It's our fake database. In our project we have users so let's add our users there.
{
"users": [
{
"id": "1",
"name": "User 1",
"age": 25
},
{
"id": "2",
"name": "User 2",
"age": 27
},
{
"id": "3",
"name": "User 3",
"age": 30
}
]
}
The most important point is that it's a json. So we need to wrap everything is double quotes.
Now we can simply start our fake json server with
json-server --watch db.json
If we open browser in localhost:3000/users we can see our created users. The most important that it's not only 1 request. We can do PUT, PATCH, DELETE, POST also to this route and json-server will update our db.json file accordingly.
Now as our fake API is ready let's jump back to our Vue project. We have all users are inside Users.vue component. Now we want to get them from API and remove the raw data.
Vue doesn't have a extension to fetch data from API inside anymore so we need to install additional library in order to fetch data. I prefer axios library which is the most popular solution on frontend to fetch data. Let's install it in our project.
yarn add axios
Now we want in our Users.vue to fetch users when our component is initialized. The is special mounted function in Vue for this.
export default {
name: "Users",
...
mounted() {
console.log("initialized Users");
},
};
As you can see in browser now every time when we reload the page we are getting this message whne our Users component is mounted to the DOM. So it's exactly the place where we want to fetch the data. For this we need to import axios and do our fetch request.
import axios from "axios";
....
mounted() {
console.log("initialized Users");
axios.get("http://localhost:3000/users").then((users) => {
console.log("users", users);
});
},
So here we are making get request to get users from our json API. In browser we can see that we are getting correct response from the backend. Now we just need to set this data inside our users property and remove raw data.
export default {
name: "Users",
...
data() {
return {
users: [],
};
},
...
mounted() {
console.log("initialized Users");
axios.get("http://localhost:3000/users").then((users) => {
this.users = users.data;
});
},
};
As you can see in browser our users are successfully rendered and we are using API call for this as in any real application. Now let's do the same with add user and delete user to implement everything with our API.
removeUser(id) {
axios.delete(`http://localhost:3000/users/${id}`).then(() => {
this.users = this.users.filter((user) => user.id !== id);
});
},
We make a delete request and after it was successful we update users list is our component.
addUser(newUserName) {
const newUser = {
name: newUserName,
age: 30,
};
axios.post("http://localhost:3000/users", newUser).then((createdUser) => {
this.users.push(createdUser.data);
});
},
Here is our addUser request. The main different is that we removed generating of ID. This is because in real API the ID of the element is being created on backend automatically so we just pass the user information that we want to add without ID.
In this video you learned how to create mock API using json server package and how simple it is to work with APIs inside Vue. It's like in the plain javascript but of course after assigning data to our property Vue does all magic with rerendering for us.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done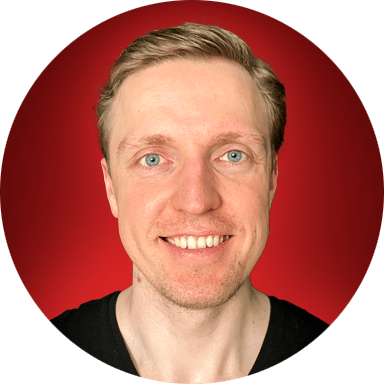