React Redux With Hooks Tutorial - Real Example
It this video we will look on React hooks which where created to work with Redux in new hooks approach.
Let's jump right into it.
So here I have a create-react-app where we already implemented connect, reducer and even selectors. If you missed previous video I will link it here on the top. So lets go through code so you can understand it better.
Now we want to refactor all this code from classes to hooks approach. Let's comment everything out and crease a functional component.
const App = () => {
}
export default App
Now the question is how we can get some properties from Redux state in the same way how to did previously. So as you can see our createStore, reducers and Provider and staying there without any changes.
To hooks to do the same like we did with connect and mapStateToProps is useSelector.
const App = () => {
const username = useSelector((state) => state.users.username);
console.log(username)
}
So we want to get username property from our state. Here we used useSelector and passes inside a function where we get a global state and we return some data. As you can see it is really similar to mapStateToProps but there are some things to know.
First of all we can return here any data. In mapStateToProps we could return only an object so than connect can compare if object changes. useSelector is being run after every render and it makes a reference comparison between previous value and new value. So if we have only single useSelector and nothing that causes rerender of the component our component will rerender only when the value of useSelector changes.
Also we don't have anything to do with ownProps like in mapStateToProps because our useSelector is directly in component and we already have access to all props.
So normally we are writing useSelector for every variable that we need.
const username = useSelector((state) => state.users.username);
const search = useSelector((state) => state.users.search);
const users = useSelector(usersSelector);
const filteredUsers = useSelector(filteredUsersSelector);
So here is some cool stuff. If you remember we wrote selectors in previous video we move selecting logic outside of our component and be able to memoize our data. As you can see our selectors are working out of the box just because it's the same function which returns a slice of the state.
Now let's add our markup back and check what else do we need.
const App = () => {
const handleUser = (e) => {
dispatch({ type: "CHANGE_USERNAME", payload: e.target.value });
};
const handleSearch = (e) => {
dispatch({ type: "CHANGE_SEARCH", payload: e.target.value });
};
const addUser = () => {
dispatch({ type: "ADD_USER" });
};
return (
<div>
<input type="text" value={username} onChange={handleUser} />
<input
type="text"
placeholder="Search"
value={search}
onChange={handleSearch}
/>
<button onClick={addUser}>Add user</button>
<ul>
{users.map((user, index) => (
<li key={index}>{user}</li>
))}
</ul>
</div>
);
};
So here the only question is how to get dispatch method. For this we have useDispatch which directly returns dispatch function.
const dispatch = useDispatch()
Now as you can see all code is working as before.
But here is an important thing about memoized selectors. Just to remind you we are using a reselect library to make our filteredUsers calculations only if array of users or search changes. For us now everything is working but if we write our createSelector from reselect inside a React component directly in won't work because reselect has a state. This is how it returns cached version. If we write this code inside a component we will get the new value every render. So the easiest way to fix this is always write reselect outside of the component. For example inside selectors file.
One more hooks inside react-redux is useStore hooks. It returns access to the whole store. Normally you should never use it because we have a nice abstraction with useSelector hooks.
const store = useStore()
So here are important points to remember.
- React hooks approach with Redux is not a silver bullet. There are pros and cons compared with connect.
- React-redux did a get job of implementing useDispatch and useSelector for us to use
- You should always check how often your component rerenders because with hooks it's much easier to have rerenders what you don't need.
- Memoization can also be tricky with hooks
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done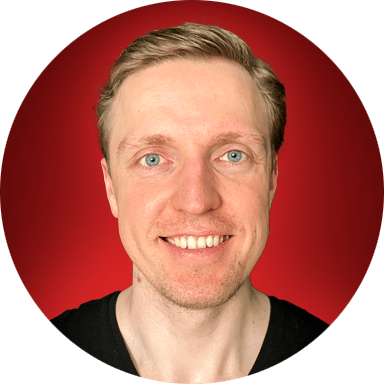