Ternary Operator Javascript
In this video you will learn about using ternany operator in javascript. It may sound like a video for beginners but there lots of advanced usages and tips so make sure that you watch this video until the end.
Let's jump right into it.
So what is ternany operator? Let's check on the example. Normally when you want to write some condition you simply use if else statements for this.
const someFn = (isActive) => {
if (isActive) {
return 'It is active'
} else {
return 'It is inactive'
}
}
someFn(true)
Here we have a function with single parameter and it should be boolean. There we have if else to write a condition and return different strings. As you can see in browser we are getting "It is active" string because we passed true inside.
We can also refactor this code to use ternany operator instead of if else. Ternary operator is simply a shortcut to if else code.
const someFn = (isActive) => {
return isActive ? 'It is active' : 'It is inactive'
}
someFn(true)
As you can see we returned here the string directly. And ternany operator consists of 3 parts: boolean at the beginning that we want to check, then goes question mark, then what we want to get back if our boolean is true, then colon and then what we want to get back if our boolean is false.
If we look in browser, this code works example the same as if else.
This is actually all the basics that you need to know about ternany operator.
Now let's take about more advanced stuff.
First of all we are in javascript world. This means everything is less strict than it should be. This is why it's possible to pass as a first parameter not just boolean variable or condition which will return boolean but just a variable. Like this
const someFn = (isActive) => {
const a = ''
return a ? 'It is active' : 'It is inactive'
}
someFn(true)
This will work example as with boolean but actually will check the presence of the variable. So it will return false in several cases: empty string, null, undefined, NaN. This is why a lot of people like to do such check to get something back if value is present. But of course it's a javascript hack and I don't recommend using it like this. For example more string language you would get error that you can pass only boolean there.
This is why to avoid bugs I always recommend to convert your variable to boolean first.
return Boolean(a) ? 'It is active' : 'It is inactive'
Now here is a super important difference between if else and ternany operator. If else is an implerative operator and ternany operator is a declarative operator without side effects. So implerative means how it should be done and declarative is what should be done. In if else you have some logic or assignments inside so you describe how you program should work. With ternany operator you have some logic and you immediately write the result to the variable. First of all it's more declarative and secondly there are no sideeffect because you don't need to define properties first if you want to assign something to them. This is why always if possible I recommend to use ternany operator and not ifelse.
Now let's talk about things that you should avoid doing.
This of all and I see it really often is packing 2 ternaries one in another.
const someFn = (isActive, isActivated) => {
return isActive ? (isActivated ? 'activated' : 'active' ) : 'inactive'
}
someFn(true, true)
This is completely unreadable and you should never write this. We have 2 ways to refactor this code. First of all - fail fast.
const someFn = (isActive, isActivated) => {
if (isActivated) {
return 'activated'
}
return isActive ? 'active' : 'inactive'
}
someFn(true, true)
Actually I recommend always trying to write your code in fail fast approach so you get out of the function as soon as possible. It will simplify your code.
The other variant is to move one of the ternaries in additional variable.
const someFn = (isActive, isActivated) => {
if (isFoo) {
return 'activated'
}
var additionalStatus = isActivated ? 'activated' : 'active'
return isActive ? additionalStatus : 'inactive'
}
someFn(true)
One more thing that you should not do is to use ternany operator as just a function call. As you understand we can ignore assigning our code to a variable. Let's create 2 additional functions and just call it inside ternany operator.
var foo = () => console.log('foo')
var bar = () => console.log('bar')
const someFn = (isActive, isActivated) => {
isActive ? foo() : bar()
}
someFn(true)
So here we just use ternany operator to call some function based on the condition and we completely ignore what ternany operator returns. Also we have side effects inside our foo and bar. You should not set any properties or write logs because the purpose of ternany operator is to be pure and just return a value. In other case it's super difficult to debug.
So this are the main points that you need to remember when you are using ternany operators. If you forget to mention something please write down in the comments below.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
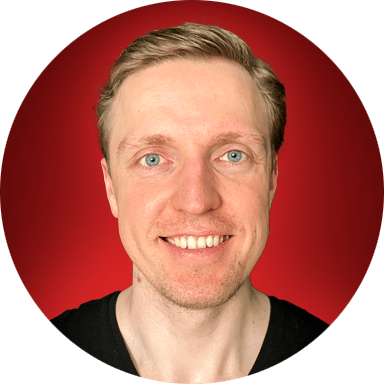