Top 5 Common Mistakes in React - You Must Know Them
This are 5 most common mistakes that people are making while creating React applications
Key (index) on arrays
The first one is using indexes for arrays when we do map. As you know you will get a warning from React if you don't write key attribute. This is happening because React needs the unique identifier for each element to rerender them correct and efficient. The most popular solution that people do is using indexes in array as keys. It may sound fine and it's kinda works. The problem happens at the moment when you will modify your array. For example you remove an element. It changes all indexes of elements and it's don't work correctly anymore. The best approach here is to use a unique identifier as a key. Usually you want to render some entities like users or posts which means the best approach here is to use their ids instead.
Not creating enough components
Problem number 2 is creating god object components or not splitting components enough. I see really often components with 500 or 1000 lines of code. This is really wrong because you pack too much business logic in a single component. It always better to have just a single responsibility inside component. When you see that you component does the layout of the page, rendering of the list, and the whole logic in every single item it's probably time to split it.
But you should also do it mindfully. It doesn't make sense to make 20 components with several lines of code each because it will be more difficult to read and support.
Passing data just to pass data
The next problem is passing data just to pass data. So normally we can store state in any of our components. It is super flexible but leads to 2 problems. First of all all our state is written all over the application and not in one place. Secondly it become difficult to pass state between components on different level and it bring the problems when you just pass data to pass data. Let's say you have some state in root component and you need this data 5 levels lower. It means that you need just pass it through 4 levels to get it.
The correct solution here is to use React context or Redux wrapper to have a global state with data that you can get on any level.
Not managing business logic
Which brings us to the next point. It is completely fine to write business logic in components and by default we don't try to move it out of components. This is not totally bad but it's better to decouple view and business logic layers. The typical solutions here are additional state management things like React context, Redux, Mobx have to solve that. Our components have no business logic and just subscribe to the data from the store. Also all business in 1 place outside of components.
Not using redux devtools
If you don't know anything about Redux you should for sure learn and understand it because it's the most popular solution for state management in React. And it has one of the best debugging tool that I saw in my life. We see our full state of application and each change that happens. And we see how our state is updated after each change. This means that sometimes you can fix a bug in your application just by looking on dev tools and how our state is changing. Because we can directly see what action made a wrong change to the state.
Dependencies in useEffect
And the most common problem that comes in React with React hooks. I think that React hooks are difficult this is why people have so many questions or problems with rerendering and performance there. And useEffect and dependencies array is the most popular place to fail. So for React we MUST define all dependencies of useEffect. So if we use some local property it should be in this array. And some people just disable a warning and not provide anything there but it's completely wrong. We should not lie to React about our dependencies. If we don't do that we can get stale information rendered. Also not all developers know that functions are also need to be defined in dependencies array but as they are created every single time we must additionally wrap them in useCallback. If we don't do that we will get an infinite loop because our dependency will change on every render.
So this were 5 biggest mistakes you must be aware of and avoid doing.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
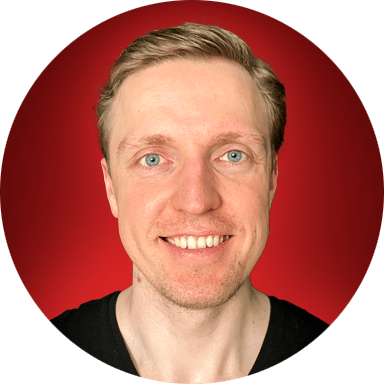