Angular Type Definitions With Typescript Interfaces
When people just start writing Angular applications it's really difficult to write correct and transparent Typescript code but this is super important. In this video you will learn how to write Angular type definitions to make your code less errors prone. I will also show you how to create Typescript interfaces and cover all your code with typings.
In previous video we finished decoupling usersList component and it's parent and it's now completely reusable. But what we are missing is some Typescript inside our code. As you saw almost all code that we wrote was mostly like writing javascript. It's not bad for start but we are writing Typescript which means that our code must be better covered with types and interfaces. Let's check on the examply why do we want that.
For now we worked with users. And for us it's just an array of objects with 3 properties. It's fine for Javascript but in Typescript we can think in types and interfaces. Actually in real application our user is not just an object. It's an entity with which we are working. So we can say "Okay in our application we have users. And we are sure that each user has only this 3 fields and they are mandatory". From javascript code all this is not clear as it's just a plain object.
Let's create an interface for our user in order to specify that this is a special entity in our application. Let's create a new folder app/types where we will store all our types and interfaces. Inside we need a user.interface.ts
export interface UserInterface {
id: string
name: string
age: number
}
So what is it? It's a special thing of Typescript which is called interface. Basically it's a schema of our User entity. Here we define what properties do we have and what types are they.
Now let's use them in app.component. If I hover the users property now you can see that it's just an object with 3 properties. Just to remind you I can see that because I have a typescript support in editor thanks to Language Server.
Now let's specify our interface here.
users: UserInterface[] = []
As you can see we used colon and our interfaces. I put square bracket after because it's an array of UserInterfaces. Now when we hover on users you can see that it's our interface.
Now you might ask why it's so important and what's the difference? First of all we can't now mess our data because we get typescript error that such field is not allowed. Secondly it's clearer for all developers when all your code in covered in types and interfaces. You just see that you get a UserInterface and return the ManagerInterface for example and not just some magic objects.
Now for example in removeUser you can also see that typescript understans and validates correct argument. Which means we can't make a mistake and use wrong property.
We can also add our interface when we create a new user.
const newUser: UserInterface = {
id: uniqueId,
name: userName,
age: 30,
}
Now we can also specify our input for users.
export class UsersListComponent {
@Input() users: UserInterface[]
...
}
And here it's much more important because if we don't specify anything it will be any. Which means we don't get any validation and it's not clear what we need to provide inside.
This is why I'm always creating interfaces for all entities that I have.
Now we can also import our Output. Because we didn't specify what type of data we are emitting.
@Output() removeUser = new EventEmitter<string>()
@Output() addUserEvent = new EventEmitter<string>()
We can do it by providing type in tags when we create our outputs. Actually EventEmitter here is a generic but it's a topic for other video. Of course all this syntax can be a bit complicated for you, especially until you get some more Typescript knowledge but it's impossible to write good Angular code without it.
In this video you learned how to write Typescript interfaces and Types in Angular.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done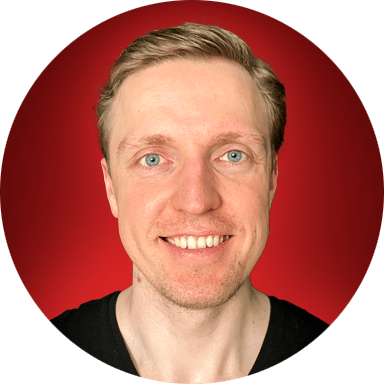