Typescript - Type Definitions Introduction
In this video you will learn how to create basic typings for variables and functions in Typescript
So here I have already setted up empty project for writing typescript. If you missed my video where we installed all needed packages and configured our project I will link it in the description.
So let's start with defining some variables. We are doing it in Typescript in the same way as in Javascript with const let and var. Let's try with const.
const hello = 'world'
So here we created a hello variable which is type string. But actually when I check a type you can see that it's not a type script but type 'world'. In this helper window we have our name on the left and after colon we have a type. And it's not a string because it's const and we can't change it's value. So it's forever type 'world'. Let's try to override a properly.
const hello = 'world'
hello = 'foo'
As you can see in console we are getting
Cannot assign to 'hellp' because it is a constant.
So this is already super useful for us. Now let's try to change the variable to let.
let hello = 'world'
hello = 'foo'
First of all you can see that it's a string now and not a 'foo' anymore. It's because we are allowed to change a value of the property but only in the string. This is why we don't get any error now. If we try to assign some other type there we will get an error.
let hello = 'world'
hello = true
Type 'boolean' is not assignable to type 'string'.
And this is the main benefit of Typescript. You can set other type inside existing types and this is why we are getting much less errors or incorrect behaviour.
Also as you can see Typescript understands and defines the correct type for each variable based on it's value. When we assign a string first time Typescript understands that it will be a string forever. We can also define a type by ourselves.
let hello: string = 'world'
let isLoading: boolean = true
To define a type we put colon after a variable and write it's type. And obviously we can't define a type string and set as a default value boolean for example.
let hello: string = true
Type 'boolean' is not assignable to type 'string'
I recommend to always define types manually. It's more visible and readable even without check each variable manually.
Now let's create some function. We can create functions in the same way as a Javascript. Using function word or writing arrow function.
const getFullName = (name, surname) => {
return name + ' ' + surname
}
This is just a normal function which concatenates 2 strings. And here we don't have any benefits of Typescript because we can pass whatever we want as arguments and then it will be wrong.
console.log(getFullName(true, ['foo']))
Obviously we want Typescript to protect us from such code. For this we need to define the types of both arguments as well as what function returns.
const getFullName = (name: string, surname: string): string => {
return name + ' ' + surname
}
console.log(getFullName(true, []))
So we define the type of each param with colon and a type. To define the type that function returns for us we write after round brackets colon and a returning type. Now we are getting a nice error
Argument of type 'boolean' is not assignable to parameter of type 'string'
So now everybody can pass only correct types inside the function which automatically protects our code from bad usage.
It's also important to mention that in this case Typescript can figure out what function is returning on it's own. But I always recommend to set it explicitly. First of all if you change the code inside maybe Typescript will change type and you won't see it and secondly it's clean from first glance what function should return.
So now let's go through Typescript notation of the function to make sure that you fully understand how it defines types.
const getFullName: (name: string, surname: string) => string
So here is our getFullName property and it's a function because you can see round brackets with 2 params and the types that are defined. After round brackets we have a arrow. This meanst that this is an arrow function and we are returning string here.
So we successfully learned how to define variables and function types in Typescript and how important it is to cover all types for Typescript correctly because in other case Typescript can't help us
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done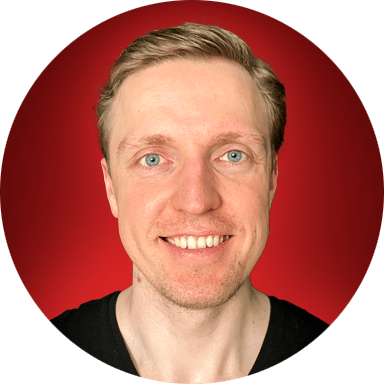