Union & Type Alias in Typescript
Hello and welcome back to the channel. In this video you will learn about type aliases and union operator in Typescript. This are basic and needed knowledge that you need in everyday work with Typescript.
Let's start with union operator. So normally we define types in Typescript with colon.
let username: string = 'alex'
This means that we can assign only strings in this property and nothing more. But what we do if we want to assign something different inside? For example number. For this we have union operator. It helps us to defined several possible types.
let pageNumber: string | number = '1'
``
And the most popular usage of union operator is some type or null. We have a property with default value as null and then later we assign there a type that we want. For example we have a field where we assign an error message. By default it's a null and if we get an error it will be a string.
```javascript
let errorMessage: string | null = null
Also you can use custom interfaces here. If you didn't see my video about interfaces don't forget to check it first. I will link it down in the description.
let user: UserInterface | null = null
But of course you should use it with caution. You don't want to end with something like this.
let someProp: string | number | null | undefined | string[] | object
Now let's talk about type aliases. So we have basic types like string, boolean, number, array, object and lots of others. What we can do is create type aliases for basic types.
type ID = string;
interface UserInterface {
id: ID,
name: string
}
Here we are defining new custom type ID which actually is just a string. Later we can use it everywhere. For example inside interfaces.
Why it is good? Because if you have in the whole applications strings and array of strings it's now that clear and doesn't force you to think in types. In this case it's much readable because we understand that this is a specific type for ID.
Let's create one more custom type.
type PopularTag = string;
const popularTags: PopularTag[] = ['dragons', 'coffee']
So here is popular tag type. It really helps to make code more understandable and readable.
So now we learned unions and we can start to use them inside type aliases.
type PopularTag = string;
type MaybePopularTag = PopularTag | null
const dragonsTag = null
And of course we can create alias types just with union of basic types
type PageNumber = string | number;
let page: PageNumber = 5
So this was everything that you need to know about type aliases and unions.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done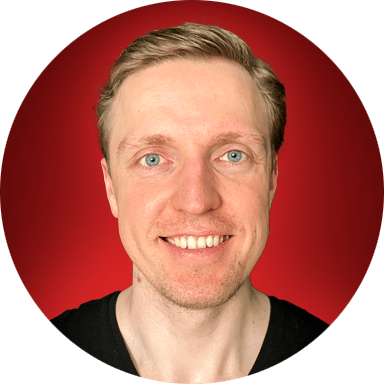