5 Must Know Javascript Concepts
This are 5 concepts that every Javascript developer should know and understand.
mutating object arrays
Most common concept and not understanding the basics is treating array and objects like primitives. People think that if they override the value it works.
const arr = [1,2,3]
const arr2 = arr
arr2[0] = "foo"
console.log('result', arr, arr2)
So here we assigned arr to arr2 and most beginners think that we just reassign the value and that it is safe to modify arr2 now without changing arr. But it works like that only with primitives where we really reassign the value of the variable. In arrays and objects we create a reference on the same object which exists in memory. Which means modifying arr2 leads to modifying arr because essentially you modify the same object in memory.
In can be even worse when it's an argument of the function. And again beginners normally think that arguments of the functions are just properties that we can override.
const basicConfig = { host: "http://google.com" };
const generateExtendedConfig = (config) => {
config.post = 3000;
return config;
};
const extendedConfig = generateExtendedConfig(basicConfig);
console.log("result", basicConfig, extendedConfig);
Here we have exactly the same problem. We pass an object as an argument and when we change it we modify object that we passed inside. But here it's even more difficult to understand the problem because we return the config and kinda assume that we returned completely other object.
What is the correct fix to such problems? Always create new objects and arrays if you don't want to modify the old one. In this case here we can return a new object, put there all values from the old object and add new value.
const generateExtendedConfig = (config) => {
return { ...config, port: 3000 };
};
As you can see we don't have this problem anymore.
Immutability
Which brings us to the second concept. It's immutability of the code. As you get from our previous example the problem that we solve was about mutable data. It means that we mutated the old object instead of creating a new one. I highly recommend to always write immutable code when possible. It will eliminated magic object changes from different places and simplifies development. What does immutability means? It means that when you use a function or do something you create a new property and never mutate an old one.
config.host = '3000'
is an example of mutable code. Using spread operator is an immutable code. In javascript lots of methods and mutable and lots of method are immutable and you need to learn what methods mutate data and avoid using them. For example even array push mutates array.
arr.push(1)
This is why it's always better to use for example spread instead.
const arr2 = [...arr, 1]
Equality and comparison
The next comment concept that you need to know is equality and comparison. And again primitives, array and objects are compared differently. You can compare primitives but you can't compare objects.
if (1 == 2) {} // correct
if ({a: 1} === {a: 1}) // wrong
Comparing of arrays and objects will always be wrong because they reference another object in the memory. So you should never directly compare objects or arrays but if you do here are 3 different ways to do it.
Compare a unique ID inside object. It makes sense when you have a list of entities and you want to compare 2 of them.
if (user1.id === user2.id) {}
You can stringify both objects and just compare strings.
if (JSON.stringify(user1) === JSON.stringify(user2)) {}
You can use some additional library which has deep compare function like Lodash or Ramda.
if (R.equals(user1, user2)) {}
It will make a deep comparing of all properties in the loop. Be aware that if you objects or array are bit it won't be fast.
Callbacks
One more basic javascript concept is callbacks. They are really everywhere. Really often we want to pass a function to other function which will call it. And it is called a callback function because we are calling it back from the place from where we provided it.
const download = (url, callback) => {
setTimeout(() => {
// script to download the picture here
console.log(`Downloading ${url} ...`);
// process the picture once it is completed
callback(url);
}, 3000);
};
const process = (picture) => {
console.log(`Processing ${picture}`);
};
let url = "https://someapi.com/someimg.png";
download(url, process);
So here we have a download function which acceppts not only url but also a callback which must be a function. Then inside we do setTimeout to emulate async call. When we done with downloading we call a callback function and pass url inside. Most important part here that download function doesn't know anything about callback at all. It just knows what arguments we must provide back. This is extremely important to a single responsibility. As you can see process is our callback function which is just a function where we get picture as an argument.
So callback is just a parameter of the function which is a function itself.
Memoization
And the last more advanced javascript concept is memoization.
Memoization is a programming technique that attempts to increase a function’s performance by caching its previously computed results. Each time a memoized function is called, its parameters are used to index the cache. If the data is present, then it can be returned, without executing the entire function. However, if the data is not cached, then the function is executed, and the result is added to the cache.
It doesn't make any sense to use it on a small amount of data. But if you do calculations with a thousands of records over and over again it makes a lot of sense to cache the results of this calculations and just return the already calculated value from cache.
const memoizedAdd = () => {
let cache = {};
return (value) => {
if (value in cache) {
console.log("Fetching from cache");
return cache[value];
} else {
console.log("Calculating result");
let result = value + 10;
cache[value] = result;
return result;
}
};
};
// returned function from memoizedAdd
const newAdd = memoizedAdd();
console.log(newAdd(9)); //output: 19 calculated
console.log(newAdd(9)); //output: 19 cached
So this were 5 imports Javascript concepts that you need to know.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
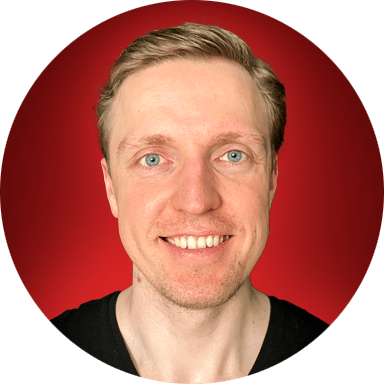