5 Must Know JavaScript Interview Questions
In this video I want to share with you the must popular Javascript interview questions that I've got during the last 10 years. Also I will share with you most important things that you should answer on this question so let's jump right into it.
Closures
The most popular question that I was asked in 99% interviews (doesn't matter at what level of knowledge it was) is "What are closures in Javascript". This is for sure the most common question in the world. So if you don't understand the closure just don't go to interview that will 100% ask you them there. As this this one of the core principles in Javascript you must in any case learn it because we use it every day.
Let's check on the example
var addTo = function (passed) {
var inner = 2
return passed + inner
}
console.log(addTo(3))
Here is a function addTo with local variable inner inside.
Now let's say that we want to remove passed argument from our function. How can we do it?
var passed = 3
var addTo = function () {
var inner = 2
return passed + inner
}
console.log(addTo())
In this case passed in declared outside and all properties which are written outside are available in our inner function.
Now let's make in a bit more difficult.
var addTo = function (passed) {
var add = function () {
}
return add
}
So here we create a function inside our addTo function are return this function. So we don't call it inside at all. Now let's try to add the same logic as at the beginning.
var addTo = function (passed) {
var add = function (inner) {
return passed + inner
}
return add
}
So here we still return add function but now it has an argument and inside we use both this argument and the argument of parent function. So actually it's the same thing like in previous variable were variable was outside of the function.
var addThree = addTo(3)
console.log(addThree)
Now after calling addTo function we get add function back. And we can call it later again. The most important part here is to check what is stored inside this function. And actually function are objects so we can check it like this.
var addThree = addTo(3)
console.dir(addThree)
As you can see in console there is property [[Scopes]] and [[Closure]] inside. There we see passed variable that we passed before. This is exactly the closure.
Normally all variables are deleted after function execution but in case with closure they are not because our child function can still use it.
Here is the short answer that I would say to show my understanding:
- Closure is an inner function inside a function which has access to the variables of parent function.
- Inner function has access to variables from parent function even after parent function was executed
- Browser keeps all variables of parent function until inner function stops to reference them
Hoisting
The next question is going in the same direction. What is global and local scope of visibility. So you need to tell that global scope is available everywhere and local scope is isolated inside the function.
Most often the next question will be to hoisting. So hoisting means that you definition bubbles to the top of the file. Var declaration and function declaration bubbles to the top. Which means doesn't matter where you create them they will be defined on the top. The most important thing here is that if you create a variable with assignment the definition will bubble but the assignment not. So at the top it will be undefined.
Here you will for sure get a question or a code example where the variable with the same name is defined inside and outside. And as vars bubbling you have difficult time in undestanding what will be the value inside. Actually it's not bad to remind the interviewer that we are writing modern javascript and write const and arrow functions which both don't bubble and problem is fixed. But if you are a junior and you want your first job it may be not the best answer from your side.
Let's check on the example
console.log(foo);
foo = 1;
-------------------
console.log(foo);
var foo = 2;
-------------------
foo = 3;
console.log(foo);
var foo;
As I said with var we have declaration and assignment. Declaration happens on the top of the file And assignments were you wrote your declaration + assignment.
So in first case we don't declare foo at all this is why at the line on console.log we will get "foo is not defined".
In second case we defined the foo later through assignment but it will bubble to the top so at the moment of console.log it will show undefined and only after it will be set to 2.
In third case foo will still bubble to the top and be assigned before console.log this is why we will see 3.
This and context
One more super popular question is this and contexts in javascript. You need to understand how this is working, that it's a reference to parent or global object, how it works in functions, arrows function, objects and classes. Actually I made a full video on that so in 10 minutes you can understand it.
Let's check on the example
const object = {
message: 'Hello, World!',
getMessage() {
const message = 'Hello, Earth!';
return this.message;
}
};
console.log(object.getMessage());
So this is example on the basic undestanding of this. In this case we work with object and this will reference on it. This is why this.message will return the message that is defined inside the object and not locally.
class Pet {
constructor(name) {
this.name = name;
}
getName = () => this.name;
getSurname = function () {
this.name;
};
}
const cat = new Pet("Fluffy");
console.log(cat.getName());
console.log(cat.getSurname());
In this case we define getName as an arrow function and not like a method of the class. You need to remember that inside arrow functions this will always be referenced on the parent. In this case it will work like a method of the class. But in the case of function it is completely different. This is function is already referenced to the global scope which is mostly useless. In our case getSurname will return undefined.
References
The next popular question is references
const basicConfig = { host: "http://google.com" };
const extendConfig = (config) => {
config.port = 3000;
return config
};
const extendedConfig = extendConfig(basicConfig);
So actually everything here is looking fine but the problem is that config that we pass is an object. And object in javascript is always a reference. Which means the config as an argument in function is a reference to basicConfig. If we mutate config inside this function it will change the property outside which is not what we want.
So solution here is to create a new Object and not modify the old one. You can do it with Object.assign or spread operator
return Object.assign({}, config, {port: 3000})
return {
...config,
port: 3000
}
How to redeclare properties in switch
switch(type) {
case "ADD":
const item = 1;
break;
case "REMOVE":
const item = 2
break;
}
This code is not valid because here it's not possible to create the same variable in different cases of switch. How we can fix that?
The problem here is that there is only one block of code. What we can do is isolate every case in new scoped block with brackets.
switch(type) {
case "ADD": {
const item = 1;
break;
}
case "REMOVE": {
const item = 2
break;
}
}
So there were 5 questions that I saw super often on interviews for any level of experience.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
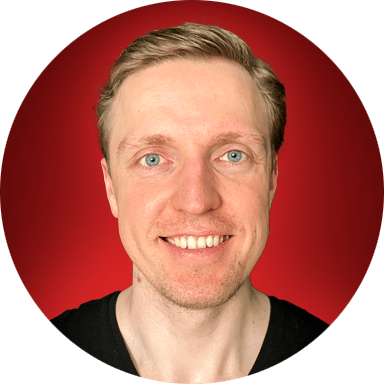