5 Must Know React Interview Questions (They Ask Them Always)
This are 5 most popular React questions that people ask on interview.
1. What are advantages of react?
The single most important question is what are the benefits of React? And this question is so important because it directly show how good you understand the ideas behind framework and what problems it is solving.
The typical and incorrect answer here is
- In React we are writing JSX it's better than html.
- React uses Virtual DOM to render the application.
Mentioning of Virtual DOM is important but it lacks the context.
Here are several important things to mention:
- Before React we typically updated DOM on our own. Bad things here is that making lots of DOM update is not fast and is difficult to support because we update DOM from all possible places with different directions of data.
- React has a concept of components tree which means that our data has single flow from top to the bottom.
- Also we don't work with DOM directly but just changing the state. What React does it simply rerender the whole tree of our application. If it does it with real DOM it would be really slow this is why it leverages usage of Virtual DOM. Virtual DOM is the representation of real DOM inside Javascript. React calculates differences from old state and new state of Virtual DOM and apply needed changes to real DOM
2. Differences between functional and class components
Next popular question is the differences between functional class components. Here you should tell that in React it is possible to create components using functions and classes. Previously we had state only in class components and functional component were completely stateless. Now it is possible to have state in functional components using React hooks. Also in class components we have life cycle methods of our component which we can use to add specific logic.
3. Differences between smart and dump component (controlled and uncontrolled)
Also really often I can hear questions regarding differences between smart and dump components. What is the difference and when to use what. So smart components are typically components with state inside. Dump components on the other hand don't have any state and just to some rendering or business logic based on the props that they received from parent component. The correct answer for that is better to you is "It depends". It should be comfortable to work with data and application. If most of your components are dump but you need to pass data through 3 levels just to keep components dump usually it's not the best architecture. But also it's not the best to subscribe a hundred of child components that we render in the loop to same data all over again because it will impact performance. It's much better to pass data from parent everywhere then.
4. State in function components, hooks
Which brings us to the state in components. How we can store state, how we pass it between components and what are the popular solutions for state management in React applications.
You should answer here that in class components we use this.state to store data inside the component and we use useState hooks for functional components. To pass data from parent to child we use either props or callback functions. As more advanced things we can use React context or Redux.
5. What is react context, how it is different from Redux
Which brings up the next question. What is React context how it is different from Redux and what is actually Redux. And this are all valid questions because state management is a huge part of building applications.
The most popular wrong answer here is "We don't need Redux anymore because we have React hooks and context". It is popular because there are lots of articles that Redux is dead and we can implement the same thing with React context. This is actually true but the point is that Redux is build on top of React context feature. And it gives you high level scalable architecture thorough actions, single global state and immutable reducers. And actually Redux is framework agnostic this is why we see Redux implementation as NgRx library for Angular and Vuex for Vue which uses the same patterns.
The most popular question about Redux is "Why reducers should be immutable"? And actually it directly shows how deep is your Redux understanding. As Redux needs to compare state after every change the only possible way to know if it was changed is to do deep comparison of all properties of old state and new state. This is super slow this is why Redux simply checks if old state and new state are referencing the same object. If not then Redux think that state was changed. This is why it is our job to always return in reducers new object.
6. How we can add type checking in React
And here is a bonus question which pop up nowadays much more than previously. How will you add type checking to React and what solutions are there?
The most popular thing for static type checking is to use Typescript with React. It is a well tested production solution. Less popular solution which was introduced long ago but never became popular is Flow. The main point that we get errors in transpile phase and not in runtime.
If we want something light just to validate our components we can use PropTypes library. It allow us to validate properties that we pass to component but it will do this checks only in runtime.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
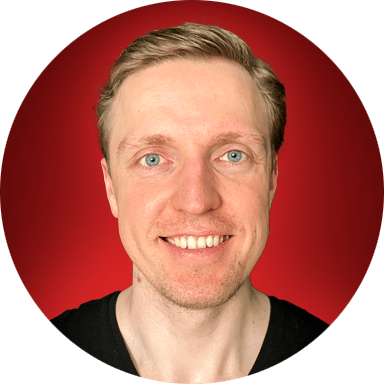