Angular Interview Questions You Should Know
This are 10 most popular Angular interview questions that you need to know in order to get position as an Angular developer and feel comfortable on interview.
How angular app is loading and working
Here you need to tell that we have an angular.json file were the config of how our application is build is written. Normally we jump to main.ts file which loads the application and provides root app.module. Inside app module we define all dependencies, routes and component which will be rendered. After this Angular renders this specific component as well as all child components as a tree.
What are advantages and disadvantages compared to other frameworks
Pros:
- Features that are provided out of the box - Angular provides a number of built-in features like,routing, state management, rxjs library and http services straight out of the box. This means that one does not need to look for the above stated features separately. They are all provided with angular.
- Declarative UI - Angular uses HTML to render the UI of an application. HTML is a declarative language and is much easier to use than JavaScript.
- Long-term Google support - Google announced Long-term support for Angular. This means that Google plans to stick with Angular and further scale up its ecosystem.
- Typescript by default - which means framework is planned for big and scalable business applications with long support time
Cons:
- Is complicated compared to other frameworks.
- Has too much to learn like RxJS, Typescript, Angular syntax, DI, etc
- The code base of the framework is much more difficult compared to React for example.
What is AOT compilation
In Angular we have 2 ways to compile our application. Just in time - which essentially means in runtime and Ahead of time - which means during the build. Angular consists of syntax what can't be executed directly in browser like Typescript or Angular templates that much be converted to plain javascript functions. We either do it in runtime while developing our application of before deploying to production with Ahead Of Time compilation.
Here are some benefits of AOT compilation:
- Since the application compiles before running inside the browser, the browser loads the executable code and renders the application immediately, which leads to faster rendering.
- In AOT compilation, the compiler sends the external HTML and CSS files along with the application, eliminating separate AJAX requests for those source files, which leads to fewer ajax requests.
- Developers can detect and handle errors during the building phase, which helps in minimizing errors.
- The AOT compiler adds HTML and templates into the JS files before they run inside the browser. Due to this, there are no extra HTML files to be read, which provide better security to the application.
What do we need modules? what is DI?
In plain JavaScript we don't have dependency injections. We just have ES6 modules with imports and exports. In Angular we have modules which are isolated pieces inside our application. We can define things like services and components inside them and by default they will be fully isolated inside this module. We can also specify what exactly we can allow to use from the module. Which means we can create a registration module and say that we want to use other module as a dependency for example user module. Only if user module allow us to access UserService inside then we can use it inside our registration module. So it's a really good way of building module dependencies in your application.
What are lifecycle hooks in Angular
Every component in Angular has a lifecycle, different phases it goes through from the time of creation to the time it's destroyed. Angular provides hooks to tap into these phases and trigger changes at specific phases in a lifecycle.
There are lots of lifecycle hooks but you need to name most used:
- ngOnInit - is triggered when our component inisialized. It's nice to do something once at the beginning.
- ngOnChanges - is triggered when our input properties changes. It's useful to make some changes if our inputs were changed.
- ngOnDestroy - is triggered when our application is destroyed. Typically we want to unsubscribe from our subscriptions here
- ngAfterViewInit - after component view and all children were rendered. It's usefull if you need to work with native DOM elements and want to wait until they will be rendered
How are observables different from promises?
This question is the basics of RxJS and streams inside Angular. Promise is called only once and we can subscribe to it with then and catch. It is typical to use promises in Javascript or React applications.
In Angular we don't need promises. We have streams which is something that can produce new values indefinitely. You can name it promise where then can be called lots of times. And stream can be literally anything. Mousemove event can be a stream, API request also or just your data storage notifying about new elements is a stream. It's a core and extremely versatile tool in Angular.
How can we share data between components?
3 variants:
- Inputs to pass properties to children and Output to pass them to parents
- Services which allow components from any level to use information inside and subscribe to service changes
- Complex state management systems like NgRx where you have a single object with all data of your application which you can access everywhere
What's the difference between component and directive?
- Components are typically used to create UI widgets / Directive is used to add behavior to an existing DOM element
- Component is used to break up the application into smaller components / Directive is use to design re-usable components
- Only one component can be present per DOM element / Many directives can be used per DOM element
- @View decorator or templateurl/template are mandatory / Directive doesn't use View
What is a purpose of async pipe
It's a default Angular pipe to get a value from the stream. It's extremely important to avoid subscribing to stream on your own and use async pipe because Angular will manage it and unsubscribe on it's own.
What is Angular Ivy
Angular Ivy is a new rendering engine for Angular. You can choose to opt in a preview version of Ivy from Angular version 8.
Generated code that is easier to read and debug at runtime
Faster re-build time
Improved payload size
Improved template type checking
And this were 10 most popular Angular interview questions
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
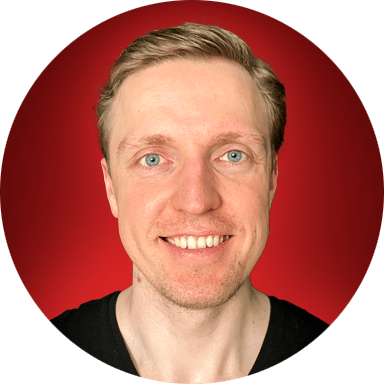