Angular Signal Output - It’s Getting Even Better
In this post, you'll discover Angular signal output and how to integrate it into your Angular applications.
With the release of Angular 17, a new feature called Angular signal input was introduced, as discussed in my previous post. This feature eliminates the need for input decorators that were previously used in Angular applications.
currentPage = input.required<number>()
Now, with input.required
, we can directly access signal inputs, making the process simpler. These local properties are signals themselves, allowing for automatic updates and providing better Typescript support.
However, the issue remains with Angular output, as there hasn't been a change in approach for handling outputs.
The latest update, Angular 17.3.0, introduces Angular signal output, completing the transition from the traditional approach to a more streamlined method. The concept is straightforward: we aim to write code for outputs in a manner similar to how we handle inputs.
// before
@Output() changePage = new EventEmitter<number>()
// after
changePage = output<number>()
The code remains remarkably similar. We define an changePage
output and specify a data type for it. This minor adjustment is the only change required, with no further modifications necessary. Output usage remains consistent, with changePage.emit
continuing to function as before.
I'm seeing the same outcome as before.
Output helpers
But that's not all as we also received two useful functions from RxJS to manage the output.
Imagine you have a stream of data inside your component, and you want to emit changes of this stream to the outside. How would you achieve that?
items$ = new BehaviorSubject<string[]>([])
ngOnInit() {
items$.subscribe(items => {
this.itemsChange.emit(items)
})
}
Previously, we achieved this by creating a subscription and emitting it with every change.
items$ = new BehaviorSubject<string[]>([])
itemsChange = outputFromObservable(this.items$)
Now, we can utilize outputFromObservable
, which performs the same task without the necessity of creating an additional subscription. It automatically generates an output that we can subscribe to.
<our-comp (itemsChange)="itemsChange($event)"/>
Now, outside of our component, we have access to this output. This approach is beneficial because it allows us to write our code in a declarative manner without needing any subscriptions.
Similarly, we can convert an output to a stream in the same manner.
changePage$ = outputToObservable(this.changePage)
ngOnInit() {
this.changePage$.subscribe(page => {
console.log('output was triggered', page)
})
}
Now, every time our output is triggered, our subscription will also be notified. These alternatives provide useful ways to work with outputs inside Angular. It creates an Observable from the output, which we can now use effectively.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done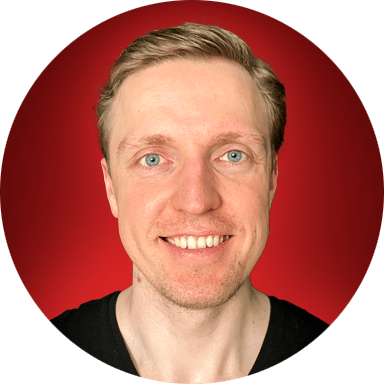