Array Iteration Methods in Javascript
In this video you will learn all methods for array iteration which are forEach, map, filter, find, reduce, some and every.
Let's jump right into it.
So normally in real applications we are working with array of objects. Why? Because it's the easiest construction to have multiple element and different information inside different elements. For example let's look on array of users.
const users = [
{
id: "1",
name: "Jack",
age: 25,
},
{
id: "2",
name: "John",
age: 30,
},
{
id: "3",
name: "Mike",
age: 22,
},
];
So our every user have 3 properties and they are packed in the array. This is the most popular construction to with with so we need to learn how to make certain operations with them.
Let's say that we want to get an array of user names now.
The first thing that people are doing in JavaScript is they learn loop like for each. And we can try to use it here.
const userNames = [];
for (let i = 0; i < users.length; i++) {
userNames.push(users[i].name);
i++;
}
console.log("userNames", userNames);
This is working fine but there are several problems here.
First problem is that we must create properties outside of for loop to save usernames.
Second problem that this code is imperative and not declarative. We don't write what we want to get but what exactly needs to be done.
Third problem is that this code is verbose. We must increase iterator every time and so on.
We can simplify this code by using forEach method to work with arrays. We can call it on existing array and as a second parameter we are getting a function where we are working with each element of the array.
const userNames = [];
users.forEach((user) => {
userNames.push(user.name);
});
console.log("userNames", userNames);
This code is much better for several reasons. First of all we don't need to manage iterator by ourselves. We just get each element from the array. Also we work directly with each element which makes code easier to read.
But we still have a problem that we write imperative code and not declarative.
So actually there is a better alternative for this function. It's a map function. It is created to go through array and return something new for each element. In our case we want to go through each user and get a username back.
const userNames = users.map((user) => user.name);
console.log("userNames", userNames);
So here we mapped through users and for each user inside we return user.name. We could return anything that we want because we are mapping old data to new data. And here is the most important thing. Map return the data back. This is why we write the result of the map directly in a variable. We should not create a variable before and the is exactly a declarative way of writing code. We define that we need to map name from each element. We don't describe how we can do it.
The next function that you need everyday is filter. So filter is need to get a new array which contains only elements that pass certain criteria.
For example we need to find all users which are older that 24. If we write this code with for or forEach but it will have the same problem.
const oldUsers = [];
users.forEach((user) => {
if (user.age > 24) {
oldUsers.push(user);
}
});
You have a specific tool to filter arrays so you don't need to go too low level and write it yourself using loops.
const oldUsers = users.filter((user) => user.age > 24);
console.log("oldeUsers", oldUsers);
As you can see the amount of code is smaller and you write the result directly in new variable. So every time when you have a goal to filter the array based on some condition you just need a filter function.
The next is find function. It works in exactly the same way like filter function but instead getting an array back we are getting the first occurrence that happened. A lot of beginners just you filter in this situation and the take the first argument of the array. This will work but the code is less readable.
const jack = users.filter((user) => user.id === "1");
console.log("jack", jack);
And here is the important difference between filter and find. Filter returns you always an array and if we didn't find anything it will be empty array.
Find result you an element of the array or undefined if nothing was found.
The next 2 methods are going together it's some and every. When you call every on the array it returns you true if all elements satisfy some condition.
const allUsersAreYoung = users.every((user) => user.age < 30);
console.log("allUsersAreYoung", allUsersAreYoung);
So here we are getting false because after checking each users age we found a user where age is 30. So not all users are young. It's a nice function when you want to check if all users confirmed emails or maybe if all products are sold.
Some function works in exactly the same way but it returns true if we have at least 1 element that fits our condition.
const atLeastOneYoung = users.some((user) => user.age < 30);
console.log("atLeastOneYoung", atLeastOneYoung);
So with the same code we are getting true because we have users who are younger than 30.
And the last method is the most difficult but versatile. It's reduce. And actually in the real code it is almost never used directly but lots of other functions are build on the top of reduce because it's so low level and flexible.
Let's say that we want to calculate total age of all our users. With reduce function we can easily do this. The idea is that we have an accumulator. So a property with some default value. Then we are going through every element and user it's data to modify accumulator. After going through all elements we are getting this accumulator back.
const totalAge = users.reduce((accumulator, user) => {
return accumulator + user.age;
}, 0);
console.log("totalAge", totalAge);
So actually it looks a bit like forEach loop but declarative. Because we define accumulator in reducer and we must return updated accumulator each time.
Also we are getting the accumulator directly back in the property so it's all handy and isolated.
The most important it that we should set the default value (in our case zero) and we must inside a function return updated accumulator. If we don't do that we will get undefined back and it's not what we want.
So this were most important functions with which you can transform data.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done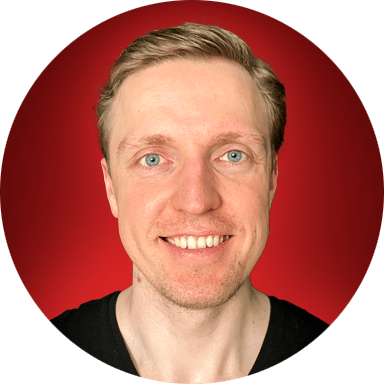