Components and Vue Files
In this video we will learn about creating components and Vue files. We will create a real example of the component that renders the list of users.
In previous video we talked about file structure in Vue project. In this video I want to talk about Vue components and how to render data inside them. So the first question is what is component as all? It's a entity inside Vue which have template, some javascript business logic and some css inside. The idea is that everything inside Vue is a component. Let's say you have an application with users list topbar, sidebar and pagination. We can for each feature create a component. One component for topbar, one for users list, one for pagination and so on. And then all our components are isolated, business logic and styles which are related to that specific feature is stored inside of that component. And actually all components are looking together as a tree. So the root component is App.vue then you render some components inside it and inside every next component we can render more and more components.
Now let's try to create a component and that we can check how it's all look like. Let's say that we need to create a users list page. As we already so in previous video we write all our route components inside views folder. So let's create there UsersList.vue. As you can see all filenames of components are written with capital letter.
The first thing that we want to do is write at least something in our template. Inside component we use template tag for this.
<template>
<div>Users list</div>
</template>
Now we need to define our component. We are doing it in script section.
<script>
export default {
name: "UsersList",
};
</script>
So here we simply exporting an object with name property. And actually our new component is completely ready. But to see it in browser we need to bind a route with our component. For this let's jump in router/index.js
import UsersList from '../views/UsersList.vue'
const routes = [
...,
{
path: "/users",
name: "users",
component: UsersList,
}
]
We need to add here new object with path, name and component. Path is our route, component is what we just created and name property we will discuss later when we will learn router deeper.
Now when we jump in /users we can see that our new component is rendered there. So it is a route component because we rendered it inside route.
So we successfully created our component and rendered there markup. But normally we want to write there some properties and business logic. As this is a users list we want to render a list of users inside our component.
The first question is how can we render some javascript inside our template. For this we can use double brackets and just write some javascript inside
<div>Users list {{ 1 + 1 }}</div>
so Vue will evaluate what is inside the brackets and render the result. It is called string interpolation. But it's a bad practice to write javascript code directly in template. Normally we just want to write there variables. So let's create a variable to render.
To define variables inside component we have specific property data.
data() {
return {
foo: "Foo",
};
},
The most important point here is that data is not an object. It's a function where we are returning an object. So here we created a property foo and we can now use it inside our template.
Now we can render it in double brackets now. As you can see in browser our property is now rendered inside the template.
Now we can do the same with users. Let's create an array of users and render them inside the template.
data() {
return {
users: [
{
id: "1",
name: "User 1",
age: 20,
},
{
id: "2",
name: "User 2",
age: 25,
},
{
id: "3",
name: "User 3",
age: 30,
},
],
};
},
So this is just a javascript array of objects.
<div>
<div v-for="user in users" :key="user.id">{{ user.name }}</div>
</div>
So here is a lot to cover. First of all we used special attribute inside div. This is how we render loops in Vue. as a value in v-for attribute we are giving user in users. Users here is our variable from data. And user is the local variable inside this div while we are going through the loop. This is why we can render name of the user inside.
Also the important thing is to add key property inside our div. We must do it every time when we are using v-for loop. Key property should be unique so Vue can render loops correctly for us. As you can see there is a special color symbol because key. We are using it there when we want to pass properties inside. As you can see user.id is a property so we need to put colon to this attribute in order to render it.
As you can see in browser, we successfully rendered the list of users inside our page.
In this video we created our new component and rendered the array of users inside it. And it's completely fine it you can't grasp all this syntax of Vue now because you will see it again and again in next videos.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done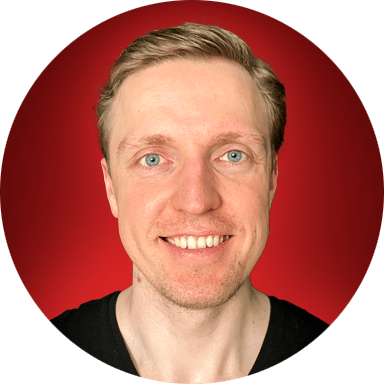