Classes in Typescript
In this video you will learn how to work with classes in Typescript. So let's jump right into it.
So here I have already setted up empty project for writing typescript. If you missed my video where we installed all needed packages and configured our project I will link it in the description.
So in Typescript as in es6 we are getting classes as a sugar for prototypes. But in Typescript they are much more powerful because we have the access to typings. Let's check on the example.
class User {
firstName: string;
lastName: string;
constructor(firstName: string, lastName: string) {
this.firstName = firstName;
this.lastName = lastName;
}
getFullName(): string {
return this.firstName + " " + this.lastName;
}
}
const user = new User("Jonny", "Muffin");
console.log(user.getFullName());
So here we can define not only properties inside class but also their types. Also in the constructor and methods we can cover everything that our class need and it types.
And there is even more. We can also use private, protected, public words like in normal languages. This makes our code really safe. If you don't know public means that it is available everywhere. Private means that you can access properties or functions only inside this class. This is nice to isolate internal functions from public API. Protected properties and methods are available inside class and child classes.
So here it makes sense to make firstName and lastName as private and only provide a public function getFullName for outside.
private firstName: string;
private lastName: string;
As you can see, now we have only access for getFullName method because it's public but not for private properties. This makes our code really safe und easy to support. But it's important to remember that all this public/private stuff exists only in Typescript. After we transpile it to Javascript there will be no validation at all.
One more thing that is nice in classes is to mark properties as readonly. If we now that it's a constant inside a class we can mark it as a readonly. Then we can assign it only once.
readonly unchangableName: string;
changeUnChangable(): void {
this.unchangableName = "foo";
}
As you can see we are getting an error that we can't modify readonly property.
Cannot assign to 'unchangableName' because it is a read-only property
The next point is about implementing of interfaces. Do you remember a video on Interfaces? If you didn't watch it go watch it first. I will link it down.
So what we can do is create an interface first and then force class to implement this interface. This is really nice if we implement a lot of same classes where we must implement some specific function
interface UserInterface {
getFullName(): string;
}
class User implements UserInterface {}
Now if we remove a getFullName function Typescript will scream because we said that User class must implement UserInterface and we didn't do that.
One more this that is possible in classes i static. When you want to write something directly to the class you can do this with static. Most often people are using it to store some constants so we can combine static and readonly here.
class User {
static readonly maxAge = 50
}
console.log(User.maxAge)
And the last thing that actually is needed is of course inheritance. We can create a child class which will extend everything from parent class. For example we have User class and we can create Admin class which will get all functionality of User but also give us a possibility to override or extends all logic.
class User {}
class Admin extends User {
private editor: string;
setEditor(editor: string): void {
this.editor = editor
}
getEditor(): string {
return this.editor
}
}
const admin = new Admin('Jack', 'Sparrow')
admin.setEditor('vim')
console.log(admin.getFullName(), admin.getEditor)
So as you can see Typescript gives us a possibility to write Javascript in more string ways like Java or C# while using classed.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done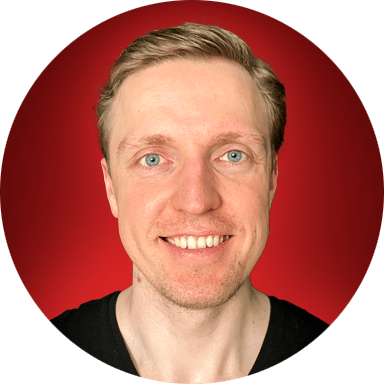