Creating Modules and Components in Angular
In this video you will learn about creating modules and components in Angular. We always want to split our application in smaller parts and in Angular we have for this 2 entities: modules and components. We will create usersList module and component to structure our application and avoiding putting too much stuff in our app.module and app.component.
In previous video we learn how Angular project is loading. And we saw the main component of the application. It's app.component. Now let's create a component from scratch, so you see how it's all working together.
Let's say that we want to render a list of users. We can't just write the whole application inside app component this is why we create new component for every scoped part of our application. And rendering a list of users sounds exactly like it.
Let's also create additional folder for our component. Because we already have to much stuff inside src/app.
Inside we need a template and a typescript file. We name them with .component by angular naming convention. Then it's clear for us that it's a component.
import { Component } from '@angular/core';
@Component({
selector: 'app-users-list',
templateUrl: './usersList.component.html',
})
export class UsersListComponent {}
As you can see our component looks exactly like a appRoot component. We have here a selector which we will use to render this component and a path to template file. One more interesting thing is that we named selector with prefix app. We are doing this to understand that this is not a library but a component of our project. Because normally in library you will have a library prefix.
Now let's just write some basic text inside html file.
Now our component is fully created but we didn't use it anywhere. Just to remind you the last file that was parsed by angular was app.component.html. Angular doesn't know anything about our new component. So we need to call it inside app.component.html
<app-users-list></app-users-list>
If we open a browser we are getting an error
'app-users-list' is not a known element
And you will see this error in the future a lot. It means that our component is not registered anywhere and Angular doesn't know how to load and render it.
To fix it we need to register our component in a module. And for now we have only app module where we can register our component. Let's do this.
To register a component inside module we need to import it and add to declarations section. As you can see we have already AppComponent there.
Now there is no error in browser and our component is rendered.
Now you know how to create components in Angular and bind them.
But there is one problem here. Just imaging that we have created hundreds components in our application for usersList, products, authentication and other features. We can of course just register them all inside app.module but it will be a huge unsupportable mess.
What we normally do is isolate a bunch of components which are related to one feature inside a module. Let's create now an additional module userList which will be responsible for our userList component. And maybe later other components which are related to userList feature.
For this let's add a module inside userList.
import {CommonModule} from '@angular/common'
import {NgModule} from '@angular/core'
import {UsersListComponent} from './usersList.component'
@NgModule({
declarations: [UsersListComponent],
imports: [CommonModule],
})
export class UsersListModule {}
As you can see we named our module also with .module.ts. Inside we have a declarations and imports arrays. Now in declarations we can declare our UsersListComponent. So we don't need to do it in AppModule anymore. Inside imports we have only CommonModule. We need to add it to every module that we create because it allowes us to write standard Angular stuff inside our module then.
Now let's just to app.module.ts and remove UsersListComponent declaration from there. Now we want to use inside our AppModule UsersListModule. In order to do so we need to import UsersListModule as a dependency of AppModule.
imports: [BrowserModule, AppRoutingModule, UsersListModule],
Now let's check if it works. As you can see we get the same error that Angular doesn't know what is usersList. Now it happens because everything is modular. UsersListComponent is registered inside UsersListModule but is not allowed to be used outside of this module. This is actually good because we have a module isolation by default.
To allow usage of UsersListComponent outside when we import UsersListModule we need to specify it in exports array.
@NgModule({
declarations: [UsersListComponent],
imports: [CommonModule],
exports: [UsersListComponent],
})
export class UsersListModule {}
Now as you can see it is working again.
In this video you learned how to create modules and components in Angular. It is looking scary and complex at the beginning but it helps a lot with modular isolation and defining the dependencies of each module.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done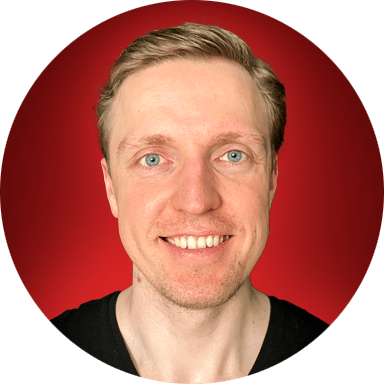