Custom Property Binding and Event Binding in Angular (with Example)
In this video you will learn about custom property binding and event binding in Angular. I will show you on the real example how to handle events in Angular and react to them inside components. We will also use property binding in order to change our input value.
In previous video we successfully rendered our list of users. It's not bad now we want more functionality. Let's say that we want to implement removing from the list.
First we need to add remove element to our markup
<div *ngFor="let user of users">
{{ user.name }} is {{ user.age }} years old
<span (click)="removeUser(user.id)">Remove</span>
</div>
So here we have new syntax. You can see that we created an attribute click inside round brackets. It's a standard notation to attach event to the element. And round brackets are super important for this. So your association in Angular should be round brackets = attaching event. Here we have a click event because this is what we want to handle. It can be change, focus, mouseover and much much more. As a value of our event we are writing a function. In our case I wrote removeUser function and passed inside user.id. Why id? Because it's our unique property inside each element and we can now inside removeUser function know what user needs to be removed. And you might think that writing round brackets will call the function directly but it's not the case with the events. It will be called only when we click.
Now let's add our new removeUser function.
removeUser(id: string): void {
this.users = this.users.filter((user) => user.id !== id)
}
So it looks for sure a bit different from javascript. Because it's typescript and here we can and want to write so many types as possible. In our case colon string means that we get as an argument string. Void means that our function doesn't return anything back. You can omit types but I don't recommend you to do so. Because typings are benefits of have Typescript and Angular and it doesn't make any sense to avoid them.
So inside this function the code should be clear. We want to remove an element from array. For this we want to filter all elements where id is not like id that we want to delete.
As you can see in browser when we click on remove button, our user is successfully removed.
This is exactly how we work with events in Angular.
Now it would be nice to make it possible to create new users. For this we need an input and a button.
<input type="text" (change)="setNewUser($event.target.value)" />
<button (click)="addUser()">Add user</button>
So here is something new. Button with click event is the same like we did with remove. But input looks interesting. As you can see we have change event inside input. It will be trigger on blur so every time when we leave the input. Inside we want to call setNewUser function and we are passing $event.target.value inside. This is some sugar from Angular. $event is a standard event what we get when we change an input. And we can use it directly in template. In our case we want to read value from the input and give it directly to setNewUser function.
So the idea is that when you finished typing inside input we want to save this text in a variable and use it later when user clicks on the add button.
newUserName: string = ''
setNewUser(value: string): void {
this.newUserName = value
}
Here is setNewUser function. We just set value that we get from the input to the property that we created. So now inside newUserName we have text that we entered.
addUser(): void {
const uniqueId = Math.random().toString(16)
const newUser = {
id: uniqueId,
name: this.newUserName,
age: 30,
}
this.users.push(newUser)
}
And here is an interesting part regarding user. To add a user we need first to generate uniqueId. Because we assume that every user has a unique id. Next we prepare a user project and simple push a user inside array.
As you can see in browser we rendered the new user. The only thing that left is age because we just put a number there but we will talk about it later.
Now the only question is to empty our input again. To do this we need to reset this.newUserName.
this.newUserName = ''
But as you can see it doesn't help with input. Because yes we cleaned the value but input doesn't know anything regarding this value. But what we can do is change the input every time when we change this value.
<input
type="text"
(change)="setNewUser($event.target.value)"
[value]="newUserName"
/>
So here is value in square brackets with newUserName. As you can see in browser our issue is fixed. Now we just need to understand what are this square brackets. So it's a value binding between a variable in class and a template. In this case every time when we change this variable in will update it here.
The only thing that is left is fixing the age property. Because for now we set it for 30 just for testing. I think that this would be a nice homework for you. Basically you just need one more input for age and you need to do the same logic with the input, like we did previously with the name.
In this video you learned on the real example how to work with events and properties in Angular. So just 2 important things to remember. Round brackets - it's event binding, square brackets it's property binding.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done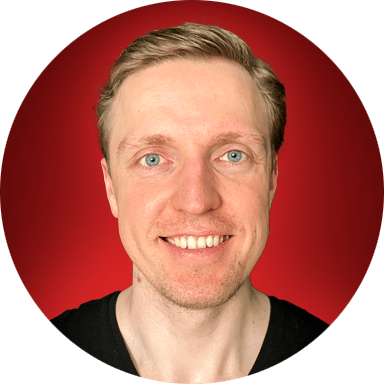