How to Generate React Application?
Hello and welcome back to the channel. In this video you will learn how to create a React application using create-react-app command line tool. This video is for you if you just start learning React and want to know the best way to create React applications.
Let's jump right into it.
So there are lot's of ways to start using React, like just adding React from CDN to your html page or download it locally. However it's not the best solution for real development. This is why we need a create-react-app. It's a CLI tool which generates for us empty React application, installs all packages and configures webserver which we run our project and reload a page on every change of the code. I see only 1 reason to use something else except of create-react-app and this is when you need to do server side rendering. But this is quite advanced and narrow topic.
So create-react-app is supported directly but Facebook so it's official and it won't just go away at some point like some small unofficial library.
The first thing that you need to do is install Node on you machine. It is recommended that you Node version should be 10 and above. So if you don't have node on your machine yet just go to nodejs.org and install it from there.
Write in console
node -v
and if you get an output then node is correctly installed.
You can generate react applications with create-react-app using npx, yarn and npm. To make it simple we will use npm because you are getting it out of the box after installing node. But here is an important note. Your npm version must be at least version 6. To check that just write
npm -v
Now you are totally ready to generate create-react-app. So you can simply write
npm init react-app my-app
where my-app is the name of the application that you want to create. So basically npm init can generate different applications for us and we need to provide the generator name inside. In our case it's react-app generator.
Now our project is created and all packages are installed.
We can jump in folder and write
npm start
to run our webserver.
As you can see in browser localhost:3000 page was automatically opened and you can see default template that was generated for us.
So actually we are done but I want to clean project a little bit so we can really write code there.
Let's remove all files from /src that we don't need any simplify our App.js.
We need to remove App.css, App.test.js, index.css, logo.svg, reportWebVitals.js, setupTests.js.
src/index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById("root")
);
src/App.js
function App() {
return (
<div>
<h1>Hello React</h1>
</div>
);
}
export default App;
Now our project is fully cleaned and we can start writing code. Also as you can see our webserver is automatically updated every time when we change the code.
I hope that this video brought you understanding how to generate React project using create-react-app. This is the most scalable way that all people are using.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done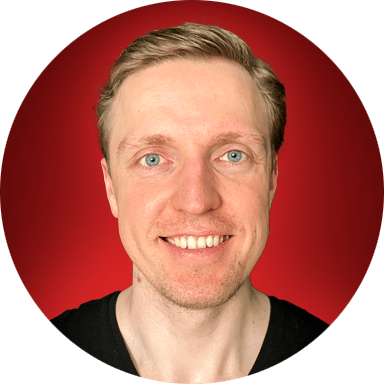