How to Install Typescript Tools?
In this video I will show you how to install everything that you need in order to start writing typescript.
First thing that we need to install is nodejs. If you don't have it on your machine yet you can just go to https://nodejs.org/en/ and download it there. To check that it is property installed you can simply write
node -v
Now we need to install typescript package globally through npm. Why globally? Because this will give us access to typescript command line tool so we can transpile our files to Javascript.
npm install -g typescript
Now to check that it is successfully installed let's you can just write
tsc --version
Now you have all tools that you need to start writing typescript. But there is one more thing. You want to see typescript errors and warning as well as check types inside your editor. If you are using some popular editor as VSCode you have this functionality out of the box. If you use some other editor or IDE you might need to install some additional package to make it working.
Now let's try to create our first typescript file and transpile it.
main.ts
javascript
const a = '1'
As you can see it looks like just normal javascript es6 syntax. I already said in previous video that browsers can't read typescript files this is why we need to transpile it back in javascript. To do this we can use tsc which is typescript command tool.
tsc main.ts
As you can see typescript created main.js for us. If we don't provide the output file in the command then our javascript file will be with the same name as our typescript file.
Let's have a look inside. So here we are getting normal javascript which can be used in browser and by default tsc transpiles code to Ecmascript 3.
Now let's make some typescript error to at least check that typescript is working.
const aaa = 'a'
console.log('aaa', aaa.foo())
So here I created a string and tried to call some method on it. In Javascript we would get a runtime error that "foo is not a function" but here we will get a transpiling error in console before this error gets in runtime.
main.ts:2:24 - error TS2339: Property 'foo' does not exist on type '"a"'.
We will talk more about types in later videos but now at least we know that typescript is working.
Also it's really time consuming to write every single time tsc when we want to transpile our code and of course at some point we will forget to do that and we will look on the previous state. To avoid this there is a build in watch in tsc command.
tsc main.ts -w
As you can see now we are in watch mode and tsc hangs there indefinitely waiting for our changes.
Now we are almost ready but I want to talk about config files for typescript. We can for sure write lots of arguments in tsc command because there are millions of configuration properties but it's a wrong way to go. We can create a config file for typescript and put there all configurations that we need. It is called tsconfig.json. So let's create it now.
{
"compilerOptions": {
"rootDir": "./src",
"outDir": "./dist"
}
}
So here I specified 2 properties inside compilerOptions. Rootdir is where all our typescript files are (normally it's a folder with your project) and outDir is the folder where javascript files will be generated.
Now we just need to create src folder and move main.ts there.
We don't even need to specify specific file with such approach because typescript will transpile all files in src folder.
tsc -w
So we successfully installed all tools that we need to start writing typescript and wrote a starting tsconfig file to simplify working with command line.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done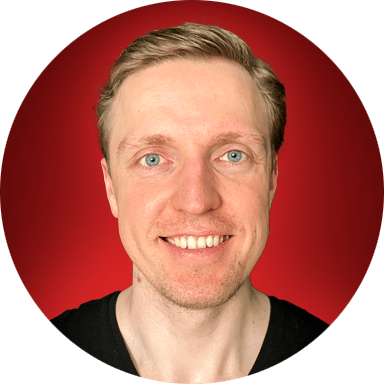