How to Structure React Applications
In this video you will learn how to structure you React applications in a way that they will be scalable and easy to support.
Let's jump right into it.
So React by default doesn't give any restrictions or recommendations about file structure at all. We just have components tree but how we structure or split our files is our own choice.
But actually in all applications doesn't matter React, Angular, Vue or even backend languages we have 2 ways to structure our code. The most important point in any of them that we have 1 entity in a single file. Sometimes it's not the case but we should keep it in mind.
The first one is by data types. So we know that we have components everywhere. So we can pack all component in components folder.
/src
/components
Login.js
Register.js
Feed.js
So actually this components are mostly route components. So for each route we have a component and a file.
But what should we do when we have sharable components between our route components? We can create common folder where we put sharable components.
/src
/common
Button.js
Select.js
/components
Login.js
Register.js
Feed.js
Actually this already looks file. And it will work for small projects. What do we do if we want to work with API and we want to decouple API logic from components? We can add api folder
/src
/common
Button.js
Select.js
/components
Login.js
Register.js
Feed.js
/api
feed.js
auth.js
If we work with Redux we can add actions, actionsTypes and reducers as 3 additional folder.
/src
/common
Button.js
Select.js
/components
Login.js
Register.js
Feed.js
/api
feed.js
auth.js
/actions
feed.js
auth.js
/reducers
feed.js
auth.js
So you got the point for each entity type we create new folder and inside everything is mostly flat.
One of the problems that I see here is that if our page is big we want to split it in different components. It's not actually reusable parts but reading them in single file is not comfortable. To solve this issue we can say that all components inside components folder should have an additional folder.
/src
/components
/login
Form.js
Login.js
/register
Register.js
/feed
Feed.js
In this case we can scale it indefinitely. And this is really nice structure for small and medium projects. If you don't want to overcomplicate things then I highly recommend you to start with such structure.
But here we have other type of structure and it fits bigger projects better. Why? Because problems with first structure start when we have too many files of the same entity. For example we have 100 files in API folder or 100 shared components. It's just more difficult to support. In this case structure by feature is actually better. So we want to group our folders not by type but by feature that they implement. It is also called like module structure because each our feature is like isolated module.
/src
/feed
/components
/api
/reducers
/actions
So we put everything that we need for this feature inside it. We also try to not make mess inside our feature folder by structuring there things by type.
One of the benefits of features is that we can remove a feature and everything that is related to it will be removed. In structure by type it won't work.
Also we can nest our feature in feature. For example we can combine login and register feature in auth feature.
/src
/feed
/components
/api
/reducers
/actions
/auth
/login
/components
/register
/components
And this gives us a lot of benefits. Because now we can share things between login and register feature without making it sharable between all features. So here is the question what we want to make sharable between features and how it should look like? Actually it can look exactly like any of our features.
/src
/shared
/components
/api
/reducers
/actions
/feed
/components
/api
/reducers
/actions
/auth
/login
/components
/register
/components
And now we can create a shared module inside auth.
/src
/shared
/components
/api
/reducers
/actions
/feed
/components
/api
/reducers
/actions
/auth
/shared
/components
/api
/reducers
/actions
/login
/components
/register
/components
As you can see we have the same structure like in shared folder on the top. Now everything looks similar and we can nest features as many times and make them as complex as we want without polluting sharable namespace.
So as you understand this two file structures are suitable not only for React but for any other type of application. But most important is that you should not overcomplicate it. If you have just 5 components and you are building todo list or something small probably you don't need any structure at all and just flat 5 components in single folder is completely fine.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done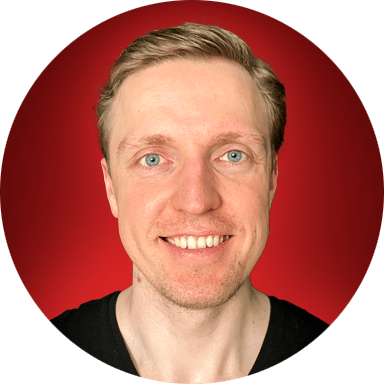