How to Use Enums in Typescript
In this video you will learn what are enums in Typescript and how they are working. So let's jump right into it.
So here I have already setted up empty project for writing typescript. If you missed my video where we installed all needed packages and configured our project I will link it in the description.
So what are enums in Typescript. It's a special feature enumerable which exists in all normally languages. It's purpose is to store a set of named constants.
Let's check on the example
For example we have several statuses and we want to use them everywhere. If we don't know anything about enums we probably create an object.
const status = {
notStarted: 0,
inProgress: 1,
done: 2
}
But with enums we can write the same code better.
enum Status {
NotStarted,
InProgress,
Done
}
let status: Status = Status.NotStarted
console.log(status)
So here we defined a status enum and used it as a type of the variable. This means that we can assign there only values from this enum. What is more important enum values can be used at a value and it will transpile to javascript and work in runtime. As you can see we are getting 0 in console. This is the same like we are getting indexed of the array. Each of values in Enum has each unique id.
Why it is better? First of all because we can use Enum as a type. Secondly we can use it as a value. And we can also mix it later with other data types for example by using unions.
But of course it's not always comfortable to work with numbers. We can also create a enum of string and mostly we are using only them because it's more readable.
enum Status {
NotStarted = 'notStarted',
InProgress = 'inProgress',
Done = 'done'
}
let status: Status = Status.NotStarted
console.log(status)
Also it's really common to use enums inside interfaces because we can be sure that the property will be only one of the enum values.
interface Task {
id: string
status: Status
}
Also I highly recommend you to postfix enums with word Enum the same way like I recommend to do with interfaces. In this case we can easily distiguish with what we are working.
interface TaskInterface {
id: string
status: StatusEnum
}
So enums are super important part of Typescript which you must understand and use correctly.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done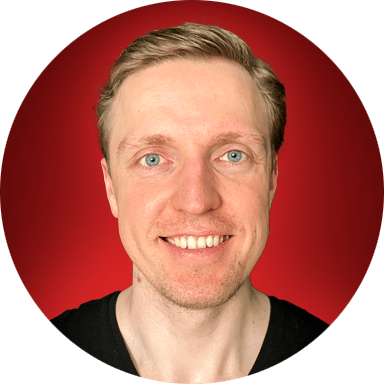