How to Use useRef in React Hooks?
Hello and welcome back to the channel. In this video you will learn one of the important React hooks and it's useRef.
Let's jump right into it.
Here I have empty create-react-app project where we can directly start writing code. If you don't know how to generate react project with create-react-app I made a video on that so go check it out first.
I you can see in browser I have just a single h1 tag and it's our App.js component. Let's have a look. So it's a normal stateless React component with markup inside.
As we are using React bigger than 16 version (actually 17) we can use hooks inside without installing any additional packages.
So what exactly is useRef hook? It's a hook implementation of working with refs in React. If you don't know mostly we are using refs to work with DOM elements, for example to make call some direct method of DOM.
Let's check on the example
import React, { useRef } from "react";
function App() {
console.log("component is rerendered");
const textInput = useRef();
return (
<div>
React hooks for beginners
<input type="text" ref={textInput} />
<button onClick={() => textInput.current.focus()}>
Focus the text input
</button>
</div>
);
}
So here we are creating a empty useRef and attaching it in the input. Now we always have a reference to this input in textInput.current. This is why we can focus the input after clicking on the button.
So this is actually the most popular way of working with reference. We simply bind them to elements and access this DOM elements directly.
But here is one bad thing that people like to write. When you work with forms you can put a ref on each element and just read direct DOM element on submit.
<button onClick={() => console.log(textInput.current.value)}>
Focus the text input
</button>
But this is really bad approach because this happens completely outside or react and there is zero reasons to do this. You can achieve the same behaviour by using useState hook. If you didn't watch my video about useState hook I will link it down in the description box below.
Let's do it the correct way.
function App() {
const [username, setUsername] = useState("");
return (
<div>
React hooks for beginners
<input
type="text"
ref={textInput}
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
<button onClick={() => console.log(username)}>
Focus the text input
</button>
</div>
);
}
In this case our component is aware of username value at any moment of time, we are not tricking react and we can do whatever we want with local state at any moment.
But DOM elements is not the only use case of refs. As you remember we normally are using useState to save a value between rerenders. We can also store any mutable variable inside ref between mutations. And the most popular usecase is to store previous value of something. Let's say here that we want to store previous username that was in our input before it was changed.
const oldUsernameRef = useRef("");
useEffect(() => {
oldUsernameRef.current = username;
});
...
{oldUsernameRef.current}
So we are creating useRef with empty string as a default value. Also we are defining useEffect here. If you didn't know what is useEffect go check my useEffect video first. I will link it down in the description.
So here we are setting in our ref a current value of username. But the main important point is that setting a ref doesn't cause rerendering. And this is the most important difference between useState and useRef value. Which means in this exact render inside DOM we see the old value and only after something retrigger rerender we will see the new value.
So it's important to remember setting a reference doesn't trigger component rerender.
So this is how you can use useRef hook. But I always recommend to minimise it's usage because it's more difficult to debug it that for example useState.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done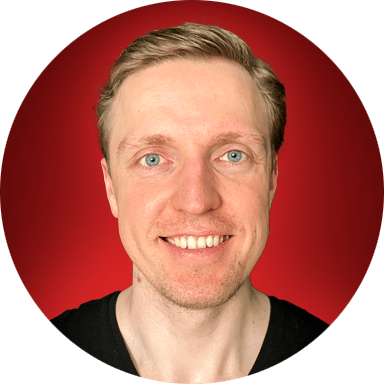