Learn Json in 10 Minutes
JSON is one of the basic concepts for any programming language. In this video you will learn what is JSON and how we can use it in less than 10 minutes.
So JSON is a special format of data which is easy to read and write and use in any programming language.
Normally we store JSON in a file with JSON extention.
JSON is a pair of key and value inside bracket.
{
"name": "Jack"
}
So here we have a key - name and value Jack.
Most common mistake is that people forget to wrap keys of the JSON in double question. Without them our JSON is not value.
We can add other keys and different types of data like strings, numbers, booleans, objects and array.
{
"name": "Jack",
"age": 30,
"isProgrammer": true,
"colors": ["black", "white"]
}
So this is an example of typical JSON. As you can see it's easy to write and read. Also this is the most common data type for communication between client and server.
For web development it's important to understand how we can work with JSON inside Javascript. So actually we can just take a JSON and assign to the variable and we get a Javascript object. Which means that Javascript supports JSON out of the box. But additionally we need to learn 2 commands to work with JSON - to parse it and to stringify. Most often we get JSON as a string from server and to be able to read it as a Javascript object we must parse it.
const str = `
{
"name": "Jack",
"age": 30,
"isProgrammer": true,
"colors": ["black", "white"]
}
`
const result = JSON.parse(str)
So we just throw our JSON string inside JSON.parse method an we get Javascript object. It's important to remember that if our JSON is invalid JSON.parse will throw an error.
To convert our Javascript object to string we can use JSON.stringify function. We typically need to do this if we want to send our data to server.
const stringified = JSON.stringify(result)
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
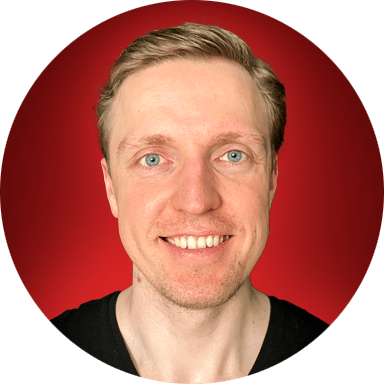