Mastering NX Angular Monorepo: Simplify Development and Boost Productivity
In this post you will learn what is monorepo and how you can setup it by using NX project.
A little bit of theory
The first question is "What is monorepo?". This is something that is mostly interesting for big applications or companies where you have lots of different projects and you somehow need to build that whole ecosystem around them.
Here you can see what is Monorepo.
Monorepo is a single repository which contains multiple projects with well-defined relationshops.
Here on the picture you can see monorepo and polyrepo. These are 2 popular ways how we are working in the companies.
First of all let's talk about polyrepo. The idea is that you have different repositories for different project. Then you need some libraries which are shared between these projects. For every single library you also create an additional repository. Every repository can do it's own releases and versioning.
Monorepo is different. Inside a single repository you have all your projects and they can work together.
Both these variants have pros and cons. It is not like one of these variants is superior.
One more really important line to remember is that monorepo and monolith are 2 different things.
Monolith means that you just throw everything that you have, all your projects and libraries in a single repository and you don't structure it at all.
Monolith is completely unsupportable. Monorepo is something completely different. You have a strict structure and strict rules how all your projects are working together.
But why do we need monorepo and not polyrepo? The main problem with polyrepo that it is really tedious as you must release in every project separately and then update all your dependencies.
Inside monorepo you have just a single repository and a single release. It is easier to make fast changes but nothing is isolated.
NX
Which brings us to NX. NX is one of the most popular solutions to create monorepo. This is exactly what we will use in this tutorial to create several projects and libraries in a matter of minutes.
NX organizes for us the whole repository with all our projects and all our libraries.
Additionally to that we will get all commands to start, build, test and lint our apps and libs out of the box.
Creating a project
In order to generate new Nx project we write
npx create-nx-workspace@latest
It installs the CLI for NX and ask us some questions about what repo to create. We want to select Integrated monorepo
as this is the easiest to start and you get all stuff preconfigured. Next we select that we want to create Angular
application. The next question is a name of our company. Let's set it to foocompany
as this is the whole repository for the whole company. Then we must provide a name for our Angular application. Let's name it shop
.And select if we want standalone components. Yes
we want.
As you can see everything is installed successfully so let's look on our project.
Most important folders for us are apps
and libs
. We create all applications with own webserver in apps
and shareable things that we want to reuse in libs
.
Inside apps
we have a shop
folder which is exactly our Angular application which was generated. This is just a normal Angular application inside.
Now let's try to start it.
npx nx run shop:serve
So all commands in NX are always run
then the name of the application, colon and the name of the command. For example serve
or build
. This will start for us an Angular project.
It doesn't really matter if you have an Angular, React or any other project. You will always use the same command.
As you can see in browser our project is running smoothly. Which means that we can create any amount of projects and they will be created in apps
folder.
Adding React project
Now I want to generate one more project but I want to generate a React project.
You want to use the same framework across the company. Obviously it is not always possible but it is really difficult to share things between frameworks.
If you have just a single framework and you share libraries between different projects which use the same framework then it becomes much easier for you to make updates or implement new components.
But sometimes it is not possible this is why let's create a React application here.
npm install @nrwl/react
npx nx g @nrwl/react:application admin --ts
First of all here we installed nrwl/react
which we need to be able to generate React projects. Secondly we generated a project with attribute -ts
. It tells NX that we want to generate Typescript project. After we get standard questions about CSS and if we need React router.
As you can see our project is generated in apps/admin
. In order to start it we can use
npx nx run admin:serve
Sharing libs
We successfully generated 2 projects and 2 different teams can implement these projects. But what can we do if these teams want to share something? This is exactly why we have libraries.
In order to generate a library we can write
npx nx g @nrwl/js:lib utils
So we generate a Javascript library with name utils
. Here I want to choose none
as a test runner and tsc
as a bundler as I want to share typescript files between these 2 projects.
Our library was created and we have it inside /libs/utils
. There is no executable for the web server as this is just a library.
Let's create a function which we can use across 2 projects.
// lib/utils.ts
export const range = (start: number, end: number): number[] => {
return [...Array(end - start).keys()].map(el => el + start)
}
Now in both Angular and React projects we can import our library and use this function.
import {range} from 'foocompany/utils'
export class AppComponent implements OnInit {
ngOnInit(): void {
console.log(range(40,45))
}
}
Now in our Angular project we get an array in our console.log.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
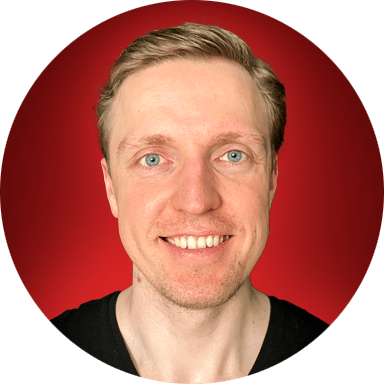