My 5 Most Used Javascript Tips - It Will Improve Your Code
Here are my 5 Javascript tips that I got during last 10 years for experience which will make your code safer and easier.
Use ternary operators
Most beginners use if else conditions a lot.
const checkActive = isActive => {
if (isActive) {
return 'active'
} else {
return 'inactive'
}
}
So here we have a function that returns us active or inactive string based on the input. Instead we can use ternary operator to write less code.
const checkActive = isActive => {
return isActive ? 'active' : 'inactive'
}
First of all it is easier to read but most importantly you don't have nesting and you directly get a result of ternary operator back. It also doesn't allow you to create variables on the fly like you can do with ifs.
Fail fast
It's also really important that you try to fail from any function as early as possible.
const checkActive = (isActive, isProgrammer) => {
if (isActive) {
if (isProgrammer) {
return "active programmer";
} else {
return "active";
}
} else {
return "inactive";
}
};
So here we have just one more nesting and our code is already difficult to read and support. What I recommend you to do is to fall out of the function as soon as possible this will minimize nesting and be more understandable.
const checkActive = (isActive, isProgrammer) => {
if (isActive && isProgrammer) {
return "active programmer";
}
return isActive ? "active" : "inactive";
};
Here we moved active programmer check outside and simplified if else to ternary operator.
Nullish coalescing operator
Really often we want to use default value if property is not defined. And most people will you here or operator.
const user = {
name: "Jack",
};
const name = user.name || "Not set";
It actually looks fine but not correct. You can get unexpected behaviour because in cases when we check empty string or 0 we will get our fallback instead of the value.
It's much better to use here check only for null and undefined because this is what we check most of the time.
const name = user.name ?? "Not set";
In this case it will work correctly in any cases.
Boolean conversion
One more popular pitfall is incorrect checks for booleans. It happens extremely often especially in if conditions.
const user = {};
if (user && user.name) {
console.log("isActive", user.name);
}
So it looks like it is working correctly but let's check what we get inside if condition.
const user = {};
const isActive = user && user.name;
console.log("isActive", isActive);
if (isActive) {
console.log("username", user.name);
}
As you see isActive property is not boolean. It's undefined. And sure logic is correct but you may have problems in such logic just because you don't convert it directly to boolean. It's always better to convert condition to boolean and avoid bugs.
const isActive = Boolean(user && user.name)
Avoid for loops
Most often to solve their problems beginners use foo loops. It's fine but the super low level, difficult to read and imperative code.
const orders = [500, 30, 99, 15, 223];
const total = 0;
const withTax = [];
const highValue = [];
for (i = 0; i < orders.length; i++) {
total += orders[i];
withTax.push(orders[i] * 1.1);
if (orders[i] > 100) {
highValue.push(orders[i]);
}
}
So here we have a list of orders and we calculate total, orders with tax, and array of high orders.
Let's try to rewrite this code by using specific functions.
const orders = [500, 30, 99, 15, 223];
const total = orders.reduce((acc, order) => acc + order, 0);
const withTax = orders.map((order) => order * 1.1);
const highValue = orders.filter((order) => order > 100);
As you can see code is much smaller, we also use specific function to solve each problem. This code is much easier to change and support.
So this were my 5 most used javascript tips to improve your code.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
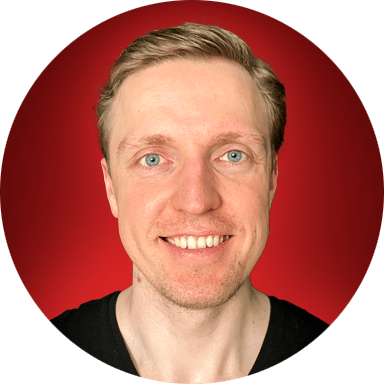