Parsing Query Parameters With Query-string Library
One of the most popular things that you need in every project is working with urls, generating them, adding query string and much more. And of course as always in plain javascript there is no good way to do that. This is why I want to show you the library query-string which solves most of this problems.
So here is the official package but on the real example it will be much easier to see benefits. Here I have index.html where I simply included this library this library.
So the most popular usecase is to get query parameters from url. For example you have a pagination in the project and you want to get query parameters in comfortable form to work with. Or sorting/filtering parameters. Normally inside window.location.search or inside frameworks which don't parse query params for you you simply get window.location.search which has string of query params inside.
The comfortable way for us to work with query params will be to convert them to object. For this we can use function parse from queryString.library.
const parsed = queryString.parse(location.search);
console.log(parsed);
//=> {foo: 'bar'}
So here we are getting an object and we can easily use properties later to build our logic.
The next usecase what we often have is to generate stringified query params. So we have several properties in javascript like order: 'asc', field: 'title', filter='foo' and we need to generate query string. Of course we can concatenate properties just inside string but it is really ugly
const stringified = queryString.stringify({order: 'asc', field: 'title', 'filter': 'foo'})
//=> '?order=asc&field=title&filter=foo'
As you can see we get fully correct query params.
And the last usecase is that you want for example get baseUrl (without query params) or you need to add some params to it. Let's make a small task what happens really often when you implement pagination.
So you can have some apiUrl. It can be just a baseUrl like '/articles' as well as url with query parameters. '/articles?author=foo'. And we want to add to this url limit and offset parameters because this is needed for our pagation feature.
As you can see we can't just concatenate a string because it's possible that you already have question mark inside and it won't be a valid string if we do that. So here queryString library solves our problems really nice.
First we can use parseUrl function from it. As a result you will get baseUrl and query params as an object. If we don't have any query params then it will be empty.
const parsedUrl = queryString.parseUrl('https://someapi.com/articles?author=foo')
Now we can add our limit and offset to existing query parameters because it's just an object
const parsedUrl = queryString.parseUrl('https://someapi.com/articles?author=foo')
const queryParams = {
limit: 20,
offset: 0,
...parsedUrl.query
}
As you can see I used here spread operator from es6 to merge in single object 2 properties and all other properties from query.
Now we can stringify query params and add them to our base url.
const parsedUrl = queryString.parseUrl('https://someapi.com/articles?author=foo')
const queryParams = {
limit: 20,
offset: 0,
...parsedUrl.query
}
const stringifiedQueryParams = stringify(queryParams)
const urlWithParams = `${parsedUrl.url}?${stringifiedQueryParams}`
As you can see without lot's of hassle, parsing a string or unreadable logic and string concatenation we in 5 lines implemented the needed logic with the help of queryString.
I'm not a fan to install libraries for every problem but queryString is super small and I use it in almost every project. So next time if you think about concatenating parameters in strings yourself just try to install this library.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done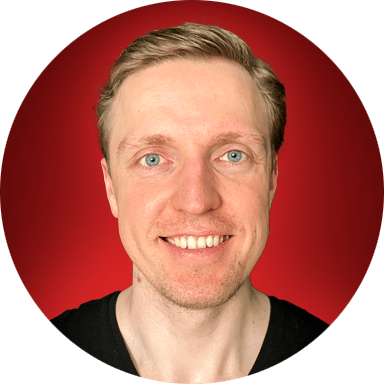