Redux Devtools vs Redux Logger (With Redux Enhancer and Middleware)
In this video you will learn about Redux devtools which is an amazing tool to fix bugs without even opening your editor.
Let's jump right into it.
So what is Redux devtools? It's a extension that can show you the whole Redux state of our application and every single action that we dispatch. The most important is that we see here the snapshot of our state befor of after action. Which means that we can just see from devtools what action updated state incorrectly without even digging deep in components or writing logs.
Let's now configure it for our project. Previously we installed Redux in React application and connected our App component to the store. If you missed that video I will link it down in the description.
The first step is to install an extension in your browser.
Then we need to install npm package of redux-devtools-extension
yarn add redux-devtools-extension
Now we need to add devtools to our createStore.
index.js
```javascript
import { composeWithDevTools } from "redux-devtools-extension";
const store = createStore(reducer, composeWithDevTools());
```
So we imported composeWithDevTools function which we call inside a second argument of createStore.
Let's check in browser how it works.
As you can see we can check our whole state here on the right and we see every action that we dispatching and it's data inside. Also we can see the snapshot of the state before and after each action.
Now the question is what is the second parameter in createStore because we never used it before. It's a store enhancer. It's a function which can add additional functionality to Redux store. This is exactly what composeWithDevTools does.
But here is one more thing that we need to learn for the future. Let's look in the package redux-logger. It is similar to redux devtools package but we don't need to install anything additionally and we get our state changes and actions inside console. It will be also useful to understand how we will install different libraries together.
So first we need to install redux-logger.
yarn add redux-logger
Then we need to import logger from 'redux-logger'
Now the most interesting but difficult part to understand the difference between middlewares and enhancers in Redux. So as I already said redux-devtools is an enhancer. Because it adds extra functionality to the Redux store. But redux-logger is a middleware. It just adds extra functionality to dispatch method. So actually every time when dispatch will be called redux-logger will log in console information about action and state.
This difference is important because as I said we pass in createStore an enhancer. So we can't pass there a middleware. So this is how we normally apply a middleware in our store.
import { createStore, applyMiddleware } from "redux";
import logger from "redux-logger";
const store = createStore(reducer, applyMiddleware(logger));
So here we used applyMiddleware middleware function where we passed logger. The idea is that applyMiddleware function returns an enhancer and we can pass a lot of middlewares inside and they all will be applied.
As you can see in browser now we are getting nice logs every time when we dispatch an action.
Now the question is how we can combine it with redux-devtools. Really easy. We just need to pass the whole applyMiddleware thing inside it as a parameter because redux-devtools understands that normally we want to configure some middlewares also.
const store = createStore(
reducer,
composeWithDevTools(applyMiddleware(logger))
);
As you can see now both redux-devtools and logger are working together.
So here are important points to remember.
- Middlewares add functionality for dispatch method and enhancers for the store
- We must use applyMiddleware in order to bring middlewares to createStore function
- Redux-devtools is amazing for debugging applications and we will use it a lot
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done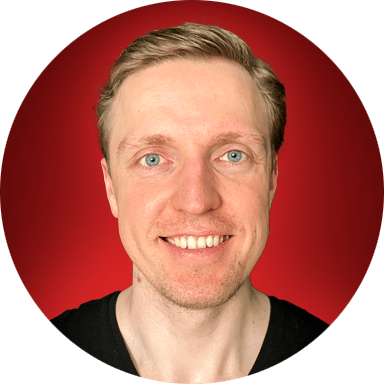