Rendering Data in Angular Templates With String Interpolation and ngFor
In this video you will learn about rendering data in Angular templates. I will should you on the real example how to do string interpolation and use ngFor loop in Angular for rendering variables from the component.
In previous video we successfully created our first userList module and component. But we wrote there only plain html. But the purpose of this component is to render the list of users. So let's do this now.
But first this that we need to learn is how to render variable from our typescript file of the component inside our template. Because all business logic of the component is inside typescript file and in html we simply rendering the data.
First let's try to add a property to our class.
export class UsersListComponent {
user = 'User for test'
}
{{user}}
If we look in browser we see that our variable was rendered. So to render a variable from our component we use double brackets in template. This thing is called string interpolation. You can write there not only variables logic like string concatenation or functions calling and so on. But I highly recommend you to keep it simple and avoid writing logic in templates at all costs.
{{1 + 1}}
Now that we know how to render a single variable in template what about arrays? Because we are doing a list of users component so we want to render array here.
First let's try to render a simple array.
names = ['Jack', 'John', 'Sam']
To render this array in template we need to use special syntax in template.
<div *ngFor="let name of names">
{{ name }}
</div>
So the whole construction star and ngFor helps us to render a list. Inside it we need to write let then some-variable of our array. Then inside of this tag we can refer to this local variable to get each element that we created.
As you can see in browser we rendered a list of names.
But normally of course we don't store just names but array of objects where we store diffent data. Let's create a users array with some information inside.
Let's create an array of users inside our component.
users = [
{
id: '1',
name: 'User 1',
age: 23
},
{
id: '2',
name: 'User 2',
age: 25
},
{
id: '3',
name: 'User 3',
age: 30
},
]
So here we have just an array of objects with id, name and age. Let's render now this data.
<div *ngFor="let user of users">
{{ user.name }} is {{ user.age }} years old
</div>
As you can see it looks exactly the same. The only difference is that we render specific properties from each object.
In this video you learned how to render data in Angular components. The most important point is to avoid writing complex logic inside your template because it's difficult to support and read.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done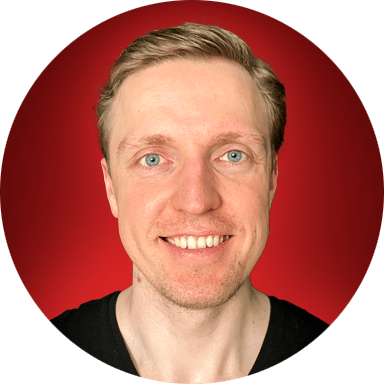