Angular 2 Routing and Navigation - Tutorial and Example
In this tutorial you will learn how angular 2 routing and navigation works. On the real example I will show you how to configure routes for our Angular application. Also you will learn what for do you need router-outlet and router-link components in Angular.
So what is frontend routing? When we load frontend application, for example Angular, then it's always the same index.html page which is completely empty. It doesn't matter in what url of the project we are going, it's always the same empty index.html. But inside this html we have javascript which decides what content to show depending on the path in url, parameters and other routing related stuff. Then when we change the page we don't reload the page. Instead javascript removes current page and renders new page. This is why it's a Single Page Application without reloading.
Angular gives us all this stuff out of the box and we can use it right away. Let's look inside app.module.ts. Inside you can see a module AppRoutingModule. Inside you can see a small module with routes on the top. Empty array means that we don't register any routes. And code RouterModule.forRoot(routes) registers this array of routes inside our application.
Normally we have a layout inside the app.component and then we loading different components on different pages. Let's try this now to see how it works. But to make it really correct we need to create 2 modules each for specific page. This is how people normally doing it in the application. Let's create 2 modules login and register.
First of all we need a login folder and a module inside
login/login.module.ts
import {CommonModule} from '@angular/common'
import {NgModule} from '@angular/core'
import {LoginComponent} from './login.component'
@NgModule({
declarations: [LoginComponent],
imports: [CommonModule],
})
export class LoginModule {}
We simple registered inside 1 component LoginComponent which is planned for our login page
Now we need to add login.component.
import {Component} from '@angular/core'
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
})
export class LoginComponent {}
and some markup.
<div>Login page</div>
The same we need to do for register page. It looks completely the same but we just replaced all names with Register.
Now we should register this two modules inside app.module.ts
imports: [
...
LoginModule,
RegisterModule,
]
Our modules are completely ready. Now we just need to create routes for this 2 modules.
app-routing.module.ts
const routes: Routes = [
{
path: 'login',
component: LoginComponent,
},
{
path: 'register',
component: RegisterComponent,
},
]
So routes is an array of objects with path and component. Now in browser we can just for example in login page without any error. But the problem is that we don't see the content of this component when we just to that page. This is because we didn't write in app.component.html special routing component which renders our components for specific pages.
<router-outlet></router-outlet>
Now in browser you can see that login page was rendered. If we just to register page than our other component will be rendered.
So this is how we normally create modules and render pages.
Let's add now 2 links inside our app.component.html so we can just without page reloading between pages.
<a routerLink="/login">Login</a>
<a routerLink="/register">Register</a>
As you can see we can now jump between pages. You might ask what is this routerLink? It's a special property binding to create links between pages. But you might ask where are square brackets? Normally we write property bindings with square brackets that's true. But if we want to simple give a string inside we can omit them. Then it will just take a string from the value on the right.
It we put here square brackets we need to define our string as a real javascript string.
<a [routerLink]="'/login'">Login</a>
And this is less readable.
In this video you learned on the real example how routing is working in Angular and what is routerLink.
As it's a last video in Angular for beginners series I think you have enough knowledge to jump in my advanced Angular course. It won't easy of course but it's the knowledge that you need to fully understand how to create applications in Angular.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done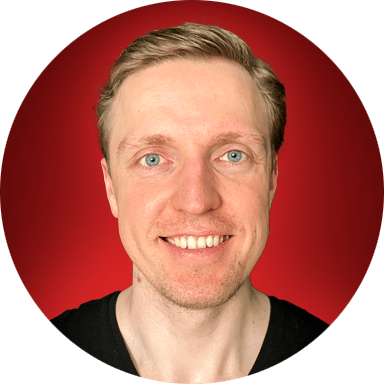