Sign-in Form Best Practices
In this video you will learn on the real example how to make a sign in form correctly to provide good user experience. So let's jump right into it.
So my first question is "do you need sign in at all"? Because the best sign in form is no sign in form. If it is possible to create you project without creating an account then it's for sure a way to go. Because with creating an account you start to store user data and you have now responsibility to protect them and store only the data which are allowed to store. So only do accounts and user management if you really need to.
Let's now create a simple form using only html elements that where intended for this job.
<form>
<section>
<label>Email</label>
<input/>
</section>
<section>
<label>Password</label>
<input/>
</section>
<button>Sign in</button>
</form>
So here are some important things to remember. This html elements that we used here are exactly the elements which were implemented to create forms. So use only them and not other elements what were not design to implement form. In this case you are using then out of the box functionality of the browser instead of implementing things yourself.
The most popular mistake that I saw is that people are not using a form and just through inputs in div and handle everything with javascript. This is of course possible but form gives you correct behaviour out of the box. For example the normal behaviour of the form is to be submitted when user hits enter in one of the input fields. And it will only work if you have a form element around.
Also a common but bad approach is to use inputs without labels and just put a placeholder inside input. This is a bad approach for 2 reasons. First, when user starts typing he doesn't see placeholder anymore and he can forget what he needs to enter. Is it an email? Or a username? Especially if you have more that several fields in form. Labels on the other case are always there and never disappear.
Secondly labels in html out of the box allow to focus on the input after you click on the label. This is really convenient on smaller devices such as tablets or phones. To get this behaviour we need to put for attribute with the ID of the form input.
<label for="email">Email</label>
<input id="email"/>
No javascript here only plain html.
Also it's recommended to place a label and an input in two separate lines and not inline. First of all it's easier to hear for the human eye and on smaller devices you can't fit 2 inline elements in any case so it makes sense to split them in two lines from the beginning.
Now let's talk about buttons. To submit a form you must use a button element. But as always a lot of people avoid doing this because it's too easy. They style divs to get a beautiful design and do other wrong things. First - always use buttons and style it like you need and secondly you should name your button in the understandable ways. It should not be names "Submit" or "Enter". If it's a sign in form then name the button "Sign in" or "login" if it's a sign up form then "Sign up" or "Register".
Also it's really important to disable submit button when we start the process of submitting form. First of all we don't want to throw too many requests in our backend when we can simply disable a submit button after the first submit. And secondly if we disable a button user can directly see that something changed. It's even better if you additionally render a small loader inside the button to show that something is happening.
Some people like to disable submit button completely until all fields are valid. It may sound like a logical step but actually it's not. The form should be responsive. User should never be in situation that he filled something not correctly and he can't proceed. He will simply close your website. Instead the form should highlight errors and point to the fields that are not filled correctly,
Now let's talk about styling. The default styling of the form is bad, small and unreadable. We must always at least increase sizes of elements and paddings between them to make this form readable.
<style>
label {
display: block;
}
section {
margin-bottom: 10px;
}
form {
font-size: 18px;
}
</style>
As you can see it's more readable than before. Also by default to have a 16px size on the desktop devices. Actually it's pretty normal for most people but I like to make fonts at least 18 px to make it bigger and more readable. Also 16px for mobile is too small for reading so you must use larger font there or your form won't be readable.
Also in a lot of popular CSS themes and UIs you can see almost transparent borders in inputs. Like they were planned to people who want to hide inputs from the users completely. Don't do that. Borders should be visible and dark enough to see them. Standard border color is completely fine actually.
The next important point is inputs validation. And here is the problem. Default validation doesn't look great no almost nobody is using it. So it's already custom javascript validation. And here we here 2 possibilities. First of all is backend validation. This is a must. Backend must validate all the data after submitting the form and show errors to the user like email is already taken or password is too short. And it's a must because backend is a single source of truth. Only by database check we can know if email is taken or not. So we can't do only client validation. First of all we don't have all information on the client and secondly people can call your API to register a user without a form. Which means backend validation for required fields or email, username validation is a must.
Javascript validation on the client on the other hand is a nice to have. From my experience I normally sticking to just backend validation where I render all errors that I got at once above the form. It's much faster then build additionally client side validation with required fields or custom validators like for email for example. But of course it's a nice addition to notify user on the fly that email is not valid.
Also the important point is that the amount of fields in the form should be as small as possible. Don't clone email or password field to confirm that user typed it correctly. Sure maybe 1% of the users will do a mistake but with enabled forms autocomplete in any of popular browsers they won't even type it. But don't forget to put an autocomplete attribute in the input for this.
<input autocomplete="email"/>
But if they do you are bothering them with additional fields. If they made mistake in password, they can always call "forgot password" functionality later. If it's a mistake in email then they won't get a confirmation letter and will just register again. So don't try to cover 1% use cases in order to ruin good UX experience for users.
One more functionality that is nice to have but not mandatory is showing a password when user clicked on "show password" button. It is consideren a good practice to allow user to check his password before registering or loginning. And it's really easy to implement it. You just need to change the type of the input from password to text and back when user clicks again.
As you can see there are lots of small things that you need to remember in order to build a good form. But most important is to keep it simple, readable, focused and responsive.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done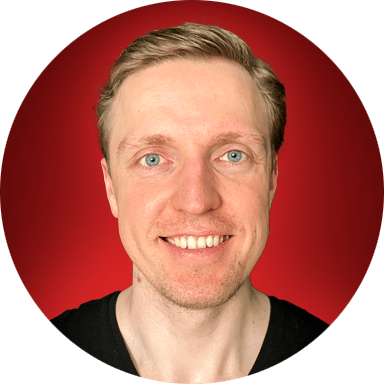