Styling Angular 2 Components and CSS Host Binding
In this video you will learn about styling angular 2 components. All styles in Angular are completely isolated in the component which means we should not care that our styles are global or how to avoid that. You will also see how Angular css host binding is working if we want to apply styles on component itself without creating additional element.
In previous video we create add and remove button to modify our array of users. In this we you will learn how to style our components on the real example. So the first question is what the difference between writing css just for any html page and Angular? The most important difference is that normally all styles are global. So it's not possible to split your styles by parts of the application using the same names. Of course there are workarounds like BEM for example which helps us with this problems.
But Angular makes things completely different. All styles that we write inside component are scoped to this component by default. Which means that class with the same name, for example list, will be completely different class in two different components.
Let's check on the example. So here is our usersList component. First of all we want to create file with styles. We can create file with any preprocessor like plain css or scss or less. For now it doesn't matter that much. Let's create usersList.component.scss.
Now we need to add this file to styleUrls inside our component.
@Component({
selector: 'app-users-list',
templateUrl: './usersList.component.html',
styleUrls: ['./usersList.component.scss'],
})
let's add some styles now.
.user-container {
border: 1px solid red;
}
Here is a user-container class with border.
Now let's attach this class to each of our users.
<div *ngFor="let user of users" class="user-container">
Looks like completely normal css.
As you can see in browser the class is now applied.
But most important for us is how the class is looking in browser.
.user-container[_ngcontent-wya-c16] {
border: 1px solid red;
}
here it's not .user-container anymore but it is scope to the special ngcontent attribute which was automatically created by Angular. This means that Angular solves for us the main problem. That we can't write the same classes all over again. Now we can create user-container in other component and there wan't be any style collisions because it's the different attribute from different component.
So we just write classes and css as always everywhere and everything is scoped.
Now let's add some css to make our users looking better.
.user-container {
font-size: 18px;
border-bottom: 1px solid grey;
padding: 10px;
display: flex;
justify-content: space-between;
}
<div *ngFor="let user of users" class="user-container">
<span> is years old </span>
<span (click)="removeUser(user.id)">Remove</span>
</div>
Now looks much better.
And here is one more important thing to notice. As you can see in DOM tree we have an additional element app-users-list. This element is exactly our component which was rendered by Angular. Sometimes we want to style exactly this element. The question is how if we don't see it in our markup and it doesn't have a predefined class?
There is a special css selector which Angular has for us example for this purpose. It is called :host.
:host {
border: 1px solid lightgrey;
display: block;
}
We can use it just like any normal class. But we need to remember that by default it's not a block element. Which means if we want to apply block styles we need to write display: block first.
In this video you learned on the real example how to work with styles in Angular.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done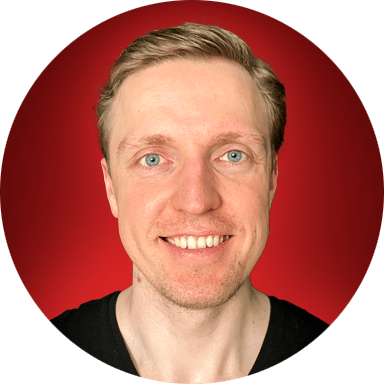