Two Way Binding and Event Handling in Vue
In this video you will learn what is property binding and event binding in Vue and how to use them.
In previous video we rendered the list of users in our new component UsersList. The easiest way to understand them is of course on the example. Here we have the list of users and would be nice to manipulate them. First of all let's built removing users. For this we need to add remove button in every user.
{{ user.name }} <span>Remove</span>
Now we want to attach a click event to this button. To do that we have a syntax v-on:click="doSomething". But people are never using it like this because there is a shortcut @click="doSomething".
So let's attach a function removeUser, where we are passinf id of the user to remove.
{{ user.name }} <span @click="removeUser(user.id)">Remove</span>
As you can see we are using @click notation to create click event binding in Vue.
Now we need to create a removeUser function inside our component. And there is a special property methods where inside we are creating all function inside our Component.
methods: {
removeUser(id) {
console.log("id", id);
},
}
Methods is an object with keys which are functions in our template.
As you can see in browser our removeUser event is triggered now and goes inside a function. Let's change our array of users now and remove a user.
removeUser(id) {
this.users = this.users.filter((user) => user.id !== id);
},
As you can see we can access our users from data property inside this. Here you use plain javascript filter in order to remove a user and reassign back in this.users.
As you can see in browser our user is successfully removed. This is exactly how we are working with events in Vue.
Now let's create a logic to add new user. For this we need an input and a button "Add".
<input type="text" />
<button>Add user</button>
What we want to do now is create a two-way data binding between an input and some property. What does it mean? It means that if we change an input our property that we binded will be updated and when we change our property input will be updated. And to do this we have v-model attribute in Vue.
<input type="text" v-model="newUserName" />
Now we need to create this property inside data.
data () {
return {
...,
newUserName: ""
}
}
Now let's render this property and see that two data binding is working.
Next we need to create a method for clicking on the button as we did previously.
<button @click="addUser()">Add user</button>
addUser() {
const uniqueId = Math.random().toString(16);
const newUser = {
id: uniqueId,
name: this.newUserName,
age: 30,
};
this.users.push(newUser);
}
As you can see first we generated here a uniqueId for our new user. Then we used a property which was binded for the input and pushed the new user in our users array.
As you can see in browser our new user way successfully rendered.
Now I want to show you how you can bind properties to the elements. We already used them actually in our for loop when we wrote colon key but I want to bring more clarity to it.
We can put a variables inside attributes of elements. For example let's say that we have a url and we want to render a href with this url.
<a :href="url">Some url</a>
So here is our syntax colon and href. With this notation we can pass variables to the Vue. Now we can just create url property inside data and it will be rendered.
But actually colon is just a shorthand as well as @ and v-on. The full notation is
<a v-bind:href="url">Some url</a>
But nobody ever write it because there is a shorthand. This is what we used in v-for when we passed key inside. We actually wanted to pass a variable and to do that we need to use v-bind syntax. And it was just a shorthand with colon.
In this video you learned a lot of stuff. How to bind properties and events in Vue, how to create two way data binding and how to define methods inside Vue components.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done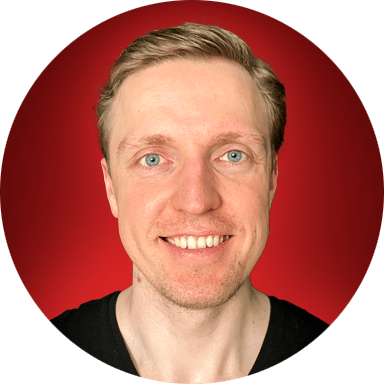