Typescript Eslint - How to Combine Them Together
In this post you will learn how you can configure Eslint together with Typescript.
Can I use Typescript with Eslint?
The first question here is if Eslint is usable with Typescript at all. In any typical project you want to use together Prettier, Eslint and Typescript.
Typescript is a really popular solution nowadays to get typings and have stricter Javascript. Which actually means before runtime in transpiling phase we will get errors from Typescript regarding our problems with typings.
We will together with Typescript use Prettier just because we want to prettify our code.
Why then do we need Eslint? Additionally to formatting code with Prettier we need to have some rules regarding our code. These are rules regarding code style that you can use in specific team.
But the main problem is that you can't use Eslint directly with Typescript.
It doesn't work out of the box with Typescript because it's parser only works with Javascript. This is why we must use additional package.
It is called @typescript-eslint/parser
and @typescript-eslint/eslint-plugin
. The main point is that we must install a parser for Typescript so Eslint can read it.
It is amazing that we can provide parser inside Eslint which allows us to work with Eslint in any language.
Here I already generated with a project with single Typescript file. Now let's install our dependencies.
yarn add eslint
yarn add @typescript-eslint/parser
yarn add @typescript-eslint/eslint-plugin
Configuration
The next step here is to configure Eslint. Typically inside our root folder we want to create .eslintrc
file. And I already prepared here a configuration.
{
"root": true,
"parser": "@typescript-eslint/parser",
"plugins": [
"@typescript-eslint"
],
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/eslint-recommended",
"plugin:@typescript-eslint/recommended"
]
}
If you used Eslint previously you typically just provide extends
with eslint:recommended
. You don't provide parser and plugins.
{
"root": true,
"extends": [
"eslint:recommended"
]
}
But for Typescript it is not enough. We must provide parser
which is exactly the package that we installed. We also provided typescript plugin here and added typescript-eslint rules in extends
section.
Now let's look on our Typescript file.
// src/main.ts
const foo = (a: number, b: string): any => {
const bar = 1;
return a + b;
};
As you can see it is not really good code. First of all we return any
as a data type here. Also we defined bar
property which we don't use at all.
But still this is a completely valid file for Typescript to transpile. But here we have problems that Eslint can show us.
For this we must define a new script which will work with Typescript.
"lint": "eslint . --ext .ts"
This command will try to read file with .ts
extension.
As you can see we get errors from Eslint. And it works only because we use this additional parser. It won't work just by default inside Eslint.
On the screen you can see that on the right we get errors from @typescript-eslint
plugin. These rules are not from Eslint but from this additional package.
Also you can see that we get warnings not only regarding Javascript code but also Typescript types. For example we get a warning about any
usage which is not recommended.
Typescript vs Eslint
As you can see we get an error that bar is assigned but never used
. And you might say here that such rules we can define in Typescript even without Eslint. Yes you can but then you can't build your Typescript code with such properties. Typically when you try to debug something it is not the behaviour that you want.
If you want to add such rule to Typescript you can write it like this.
{
"compilerOptions": {
"noUnusedLocals": true
}
}
Eslint shows us warning but doesn't stop us from transpiling code.
This is why it is much better to define such rules in Eslint than in Typescript.
Where are rules?
Your last question is for sure "Where can I see all rules for Typescript?". Here is the official website and here you can see all these rules.
If you just started writing Typescript I highly recommend you to enable Eslint which will help you a lot to improve your code.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done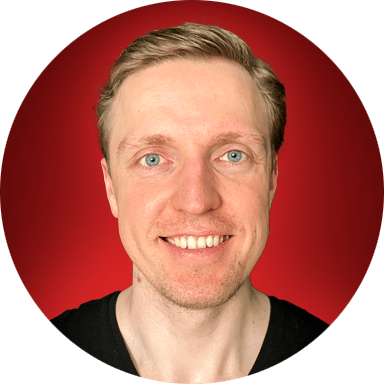