25 Coding Terms for Beginners
In this post we will look on 25 most popular programming terms that you must use when you are talking to other developers especially in the interview.
Why use coding terms?
And you might ask why do you need that at all? Is it not enough to just write code. But it's not only about writing code, it's about telling something about programming to other people. And it is especially important in the interviews where it is mandatory to use the correct terminology. If you use wrong terms when you describe something you won't be treated as an advanced developer. This is why you must understand and use these terms.
Argument
So what is argument? Argument is the word that you want to use when you want to provide some data inside a function.
const foo = (bar) => {}
For example here we have a function foo
and we are providing inside as an argument bar
. So bar in this case is an argument. And also it is allowed to call it not an argument but a parameter or param of the function. It is also completely valid.
Operator
What is operator?
const c = a + b
Typically we are writing code like this. For example we have a variable c
and we want to assign there a + b
. In this case this plus sign is exactly an operator.
And there are different operators inside all languages. Typically we have there math operators like add (+), subtract (-), multiply (*) and divide (/). But we also have an operators of comparison. For example we can also write here a < b
or a > b
, or a >= b
, or a === b
. These are all operators of comparison.
Assignment operators
But it is not all. We also have assignment operators.
let a = 1
a += 1
a -= 1
a *= 1
a /= 1
Let's say that we have here a variable a and assign it to 1. Now here we can write a += 1
. This is exactly the same like writing a = a +1
but here we used what is called assignment operator. And here we have 4 of them we have plus equal, minus equal, multiply equal and divide equal. These are all assignment operators. And actually we are not using them that often and typically you will see in the code just addition operator with plus equal sign.
Statement
And what is statement?
const c = a + b
Statement inside any language is just a single line of your code. But here I can also write console.log and it is also a statement which actually means a single line of any code is a statement.
console.log('foo')
Declaration
So, now we know what is statement. But what is declaration? Actually declaration is a statement where we declare something.
let c;
This is a declaration and an assignment. And fully it will be called a declaration statement. So, what is declaration? This is just a process to define a variable, so we can use it later. And our language knows that these variables were defined.
Expression
The next thing that we have is expression. And people are typically mixing expressions and statements. And as we already know statement is any line of the code it can be really whatever. But what is an expression?
const a = b + c
Actually expression is a statement but it must return a value. This is why this line is a statement. And this is an expression. So, the main rule here that expression will produce a value. But typically programmers use words expressions and statements when they just want to say that there is a single line and it does something.
Conditional Statements
But what are conditional statements? We already know what is statement. Conditional means that we are writing statement with some condition. And typically for example in JavaScript we will use for this if operator.
if (isActive) {}
If is a conditional statement because here we are writing a condition.
const c = isActive ? 'active' : 'inactive'
And it is not the only possibility to write a condition we can also use for this ternary operator. For example we have c
property and we are checking here isActive
condition when yes then we assign here active in other case we assign here inactive. And actually this is also our conditional statement because we are using here a condition.
IDEA
The next question is what is IDE? And actually this is integration development environment. And the most popular example of it will be IntelliJ IDEA which is a paid IDE to developed code in different languages.
So the main question here is what is the difference between just an editor like for example VSCode and IDE for example IntelliJ IDEA. And actually the difference nowadays are super small. Typically we define editors like just a place to write code but the IDE a place where we can not only write code but where we have advanced tools like autocomplete, checking our code, writing compilers and much much more. But nowadays a lot of editors for example VSCode also have inside them all these tools built in. So, nowadays editors and IDEs are super similar sometimes.
Compiler vs Transpiler
The next 2 questions are what is compiler and what is transpiler? Because actually we are interested in the difference between them.
Typically when we are using word compiler we mean that we are compiling our program in another language. And we want to say here that this code will be readable by computer. As you can see here I have GCC which is a compiler for example for C++. And it compiles our applications in binary executable code.
But on the other hand when we are talking about transpilers it is different. Yes, we also do something with our source code and we convert it to other language. But typically we are converting our code in another human readable language not computer readable language. For example the most popular transpiler for JavaScript is TypeScript.
And actually we are writing our TypeScript code and then we transpile it to JavaScript so we can run it in the browser. Which actually means the main difference between compiler and transpiler that with compiler we are transpiling something for the computer readable code but transpiler transpiles it to the same human readable code.
Binary numbers & bits
The next 2 terms that you must understand are binary numbers and bits. Binary numbers are numbers that are just created with 1 and 0 and this is the numbers how computers are reading them.
110100
So, actually all our information that we are writing, computers read as a binary system. And here you can see the typical example of the binary number. As you can see here we have just 1 or 0 and no other numbers. But what is bit? Bit is just a single letter inside a binary number. In this case the first one here is a bit.
API
The next question is really a tricky one. Because this is the question what is API? And this is the term that you can hear really a lot. And typically all developers when they are talking about API they are referring just to API that you are getting from the backend.
For example as you can see here I opened the web site and we are making API requests to conduit.productionready.io/api. And here we are getting back some articles. And typically when programmers are talking about API they are talking about backend API which delivers some data to the client. And actually this is not totally correct but API is not just this.
API is really any programming interface that you can imagine.
fooService.bar()
For example here we write that we have fooService
and this is just some service that we created and actually this service also has an API. It can be public API, private API but any methods that we are calling here or any properties is an API. So, actually this bar method is exactly the part of our API that we defined for our service. So, actually API is a way to define your functions, your classes, your applications everything which exists inside programming.
CLI
But what is CLI or command-line interface? And actually this is just a user interface which is based on text. And typically when we are referring to CLI tools we are referring to the tools that we will use inside terminal. And here you can see the example of the terminal and I can write some commands. And this is exactly the example what is CLI.
CI
Another popular term is CI or continuous integration. And actually here we are referring to some tool where inside different developers can merge their code, run different commands or programs which will check their code, run tests or deploy this code.
Which actually means this is a set of tools which is used to do some continuous things with your code.
And typically big companies like GitLab or GitHub have inside CI which actually means you can configure what will happen with your project every single time when you create for example pool request make a commit or push your changes.
Library vs Framework
The next 2 terms are library and framework. And actually when we are using word library we are talking about something where we have just a set of functions that we can use. And actually it is super misleading because when we jump for example inside React web site we see here that it is JavaScript library for building user interfaces.
But realistically React is a framework. Because actually it is super similar to Angular and View and they are also frameworks. But when we look on Lodash for example this is really a JavaScript utility library where we have lots of different methods that we can use.
Because actually inside library we don’t have anything except of some functions. And Lodash is a great example of the library we just have a bunch of functions and we can use them. This is a library.
But when we are getting some architecture like for example inside React or inside Angular when you have some rules and you have some skeleton which you can use to build your application this is not a library anymore. This is a framework.
Runtime
Another question is what is runtime? And actually as you can understand from the name this is the time while your program is running. And it is super understandable in the other languages. You simply execute some file and this file starts running and at some point it finishes.
But for example in JavaScript world we are using this term differently. When we are using word runtime
we are referring to JavaScript which is being run inside browser. And typically the whole website is running inside browser with JavaScript every single time when we load the page. Which actually means every single time when we reload the page we have a runtime because JavaScript executes everything, parses our file and this is the runtime of JavaScript.
Class, instance, method
Now we have 3 different terms that you must master. Because actually in every single interview people are mixing these words and then you understand that these people are just beginners. And these words are classes, instances and methods.
class User {
getFirstName () {}
}
const user = new User()
So, when we are talking about classes we are using class
keyword. For example here we have our class User
and this is the class.
And after this we have a user
and here we are using word new and we are creating an instance of the class. And it is super important to not refer to this user as to a class. Because it is not a class it is an instance of the class.
The next problmem is functions. All functions inside a class are methods. And people are typically using word function because they are creating a function inside class. This is actually totally fine. But the more correct term is to use word method
. For example inside user we have getFirstName
and this is the method. You can of course say that this is also the function but inside class it is a method, user is our instance and here we are calling user.getFirstName this is the method of our class.
Iteration
The next important term is Iteration. And actually iteration is just a single run through something inside your code. Typically you will hear word iteration in several ways. For example when we are talking about loops.
[1,2,3].forEach((el) => {
console.log(el)
})
Here we have an array 1,2,3 and we are using forEach loop. And inside we are doing something. Then every single time when we are making a loop is called iteration.
But it is not the only usage. For example when we are talking about frameworks and framework needs to rerender the whole tree of our components. It is also called iteration.
Iteration is just a single pass through something inside programming.
Keyword
The next term is Keyword. And actually keywords is all words which are reserved inside your language.
if for let const else
For example if we are talking about JavaScript then words like if maybe while for else they all are reserved. You can’t really define such variables because these are reserved keywords.
Middleware
Another important term is Middleware. And actually you can guess it from the name, it is something which is staying in the middle. For example we have our client on the left and we have a middleware and we have our cloud on the right. Which actually means our client communicates with our cloud.
But there is a middleware between them which actually means client sends a request to our cloud. And actually our middleware might check this request and do something with it. For example we can check if the user is correctly authenticated and if not then we should not hit cloud at all. In this case we can simply say user is not logged in. And if everything is fine and middleware did what was needed then request simply goes to our cloud directly.
Which actually means middleware is simply some code which is written between 2 different places.
It can be for example backend and frontend it can be different functions inside your own application.
Wrapper
And the least term in our list is Wrapper. And actually what is wrapper? This is something which wraps our code. And the main idea is that we have some code that we want to wrap so nobody sees this code inside. And this is super useful when we are talking about some libraries or some functions or services that you want to hide. Which actually means you are using some library but you don't really want to use functions from this library inside your application.
import _ from 'lodash'
const range = (a, b) => _.range(a, b)
If you wrap this library with your own implementation then you don't have any problem of swapping this library to another one. Because you simply need to change it in just a single place and not in the all application. Which actually means we are using wrappers when we need to hide some logic inside our code.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
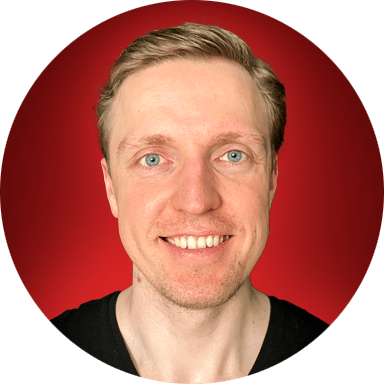