Angular 16 Features With Examples - You Must Know That
Angular 16 was already released 6 months ago and quite often you can hear questions on the interview about latest Angular features. It is a great question which shows if person knows how framework is evolving and what new features we get.
This is why in this post I want to share with you most important features from Angular 16.
Angular signals
The most important feature of Angular 16 is for sure Angular Signals.
Angular Signals is in developer preview which means they are not production ready.
In Angular Signals we get several new methods like signal
, effect
and computed
.
export class AppComponent {
title = signal('')
}
To create a signal in our component we use signal
keyword and provide a default value inside.
<input type="text" [value]="title()" (keyup)="changeTitle($event)"/>
{{title()}}
We can then render it in html just with round brackets and we can later update our signal. There are several ways to do that.
this.users.set([{id: '1', name: 'foo'}])
Set will override all data in users signal.
this.users.update(prevUsers => {
...prevUsers,
{id: '1', name: 'foo'}
})
Update allows us to use previous value to create new value. Update method is immutable.
this.users.mutate(currUsers => {
currUsers.push({id: '1', name: 'foo'})
})
Mutate allows us to just mutate existing data without returning new data.
usersChangeEffect = effect(() => {
console.log('users changed', this.users())
})
Additionally we got effect
which allows us to trigger some logic when our signal changes.
usersTotal = computed(() => this.users().length)
The last method that we got is computed
which creates new signals based on existing signals.
Signals are extremely important for performance because they work outside in the digest cycle.
Angular doesn't really know what was changed. It rerenders the whole application from top to bottom. It is not effecient this is why we got signals.
But you must remember that Angular Signals are in development preview. Yes, you can try then, you can use them inside your applications but you must be aware that their API can be changed later.
But I don't think that signals will be completely removed as they are quite awesome.
Angular enhanced hydration
The next important feature is enhanced hydration which is also in developer preview.
If we render our Angular app on the server in generated markup only once and on the client it ignores it completely and just build everything from scratch.
Not with enhanced hydration it works differently. Angular uses it's markup which was build on the server to render client markup and change it later.
You don't need to change anything or update your code. It will simply work like this internally after you upgrade to Angular 16.
ESBuild and Vite
Another important feature is a developer preview of EsBuild and Vite. Angular CLI now has a possibility to use ESBuild as a build tool which makes our building time much faster.
ESBuild is an extremely popular tool to build different languages and frameworks.
Also Angular team is looking to integrate Angular with Vite which is an extremely fast web server and project generator. For now you can generate plain Javascript, Typescript, React, Vue applications with Vite in a matter of seconds.
"build": {
"builder": "@angular-devkit/build-angular:browser-esbuild"
}
To use esbuild the only change that we need to do is to update builder
line in angular.json
. But you must remember that this is experimental developer preview.
Standalone
Another important feature is related to scaffolding of standalone applications.
ng new --standalone
When we use ng new
we can add the attribute --standlone
to generate our application without any modules but just with standalone components.
But I really like to use this command through npx which allows me to easily use any Angular version.
npx -p @angular/cli@16 ng new foo -- standalone
It will install the correct Angular CLI and generate a new standalone project.
Required inputs
Another important feature is required inputs.
export class ColorPickerComponent {
@Input({required: true}) defaultColor: string
}
It allows us to mark our inputs as required and we will directly get a Typescript error if we didn't provide an input in the component.
Miscellaneous
It is also important to remember that Angular 16 requires Node at least version 16 or 18.
I highly recommend you to switch to Node 18 because it is already LTS
We also have several deprecations. The most important is that you class approach to write resolvers and guards is deprecated and we must write functional approach instead.
Previously we wrote our code like this.
export class AuthGuardService implement CanActivate {
constructor(
private currentUserService: CurrentUserService,
private router: Router
)
canActivate(): Observable<boolean> {
return this.currentUserService.currentUser$.pipe(
filter(currentUser => currentUser !== undefined),
map(currentUser => {
if (!currentUser) {
this.router.navigateByUrl('/');
return false;
}
return true;
})
)
}
}
Here we have a deprecated approach with class AuthGuardService
which implements canActivate
method.
Now we must write it differently
export const authGuard = () => {
const currentUserService = inject(CurrentUserService);
const router = inject(Router);
return this.currentUserService.currentUser$.pipe(
filter(currentUser => currentUser !== undefined),
map(currentUser => {
if (!currentUser) {
this.router.navigateByUrl('/');
return false;
}
return true;
})
)
}
The logic is exactly the same but now we implement a functional function which must return an observable or boolean.
This is extremely important to refactor in your code as class approach is deprecated and will be removed soon.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
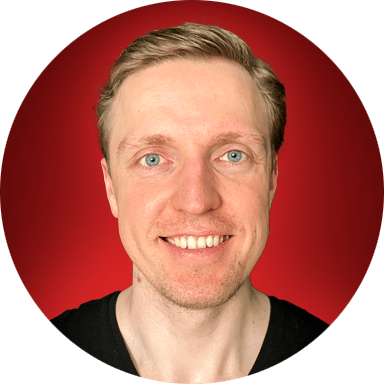