Angular Required Input - It Is Still Bad
In this post you will learn how to create required inputs inside Angular and why they are still not good enough.
Starting from Angular 16 we are getting required inputs. Essentially this is exactly what we wanted. We want to define what inputs are required for our components and get Typescript errors. But actually this new features is not implemented in the best possible way that everybody would like to have.
But before I will show you required inputs in Angular I want to show you how much better inputs in Typescript are working inside React.
Required inputs in React
In React we are getting required inputs in a perfect way. All components inside React are just functions.
const Button = ({text = 'submit', icon: string}): JSX:Element => {
return (
<button>
<span>{icon}</span>
{text}
</button>
)
}
const App = () => {
return <Button text="foo"/>
}
Here we always are getting out of the box an error from Typescript that property icon
is missing in usage of Button
. It works out of the box because these are just functions.
Required inputs in Angular
Here is exactly the same example in Angular
<mla-button text="foo"></mla-button>
export class ButtonComponent {
@Input() text = 'submit'
@Input() icon: string
}
Here we defined 2 inputs with text
and icon
but provided just text
in the component.
Starting with Angular 16 we can tell Angular that our inputs are required.
export class ButtonComponent {
@Input({required: true}) text = 'submit'
@Input({required: true}) icon: string
}
Now we are getting a Typescript error that we didn't provide an icon inside our component. This is really awesome.
But what is not awesome is that we are getting one more error.
It says that we must set a value for our icon
as it can be undefined. But it doesn't make any sense because we said that it is required.
Required still plays bad with Typescript as we get an error that it requires default value.
@Input({required: true}) icon?: string
This code doesn't make any sense because we must handle all undefined
cases in the component now.
The only fix which sounds okay is to tell Typescript that we are sure that this value is provided
@Input({required: true}) icon!: string
This is how most people use required inputs nowadays. It is fine but I don't like to use exclamation mark as something like this must work really good out of the box.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done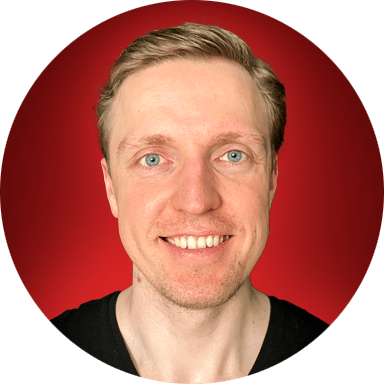