Build React Pagination Component | React Table Pagination
In this video I want to show you how you can build yourself pagination inside React. And no, you should not look for libraries. It is not that difficult and you can implement it in 10 minutes.
Initial Project
So here I already have a generated React application with an empty App.
const App = () => {
return (
<div className="container">
</div>
);
};
export default App;
What do we need to render inside and how it will work? I think that pagination is fine to just be a single component with some properties.
First of all let's create a state outside of pagination which will store current page.
const App = () => {
const [currentPage, setCurrentPage] = useState(1)
};
Now let's pass all needed properties to our future pagination component.
<div className="container">
<Pagination
currentPage={currentPage}
total={500}
limit={20}
onPageChange={(page) => setCurrentPage(page)}
/>
</div>
Here we pass currentPage
to know which page to highlight, total
amount of elements and limit
to know how many pages to render and onPageChange
which we will call inside to select other page.
This is all props that you need in order to build pagination.
Typically total will be dynamic parameter which we get from backend API request.
Styling
For our pagination component I already prepared CSS. You can take it here. This are just global styles for .pagination
, .page-item
and much more.
To apply styles you need to inject them in index.js
.
import ./pagination.css
Creating pagination
Now let's create basic pagination component.
// src/Pagination.js
const Pagination = ({ currentPage, total, limit, onPageChange }) => {
return (
Pagination
);
};
export default Pagination;
As you can see our component is rendered in browser.
Calculating pages
The next step will be to know how many pages we have in total.
const Pagination = ({ currentPage, total, limit, onPageChange }) => {
const pagesCount = Math.ceil(total / limit)
...
};
Why we do it like that? Total is for example 500 and our limit is 20. Which means we will get here a number of pages. And we are using here Math.ceil
to make our number bigger. If we have 501 items this means that we need to create one more page.
As you can see we get a correct pages count.
Rendering pages
Now we want to render all our pages starting from 1 to 25. The only way to do that is to map through the array inside React template. But in order to do that we need to create an array on numbers from 1 to 25. There is no such function in Javascript to generate an array from one number to another.
This is why we must build additional helper in order to do that. Typically this helper is called range
.
// src/Pagination.js
const range = (start, end) => {
return [...Array(end).keys()].map((el) => el + start);
};
We created here a range
function which gets start and end numbers. It generates exactly the array that we need.
Now let's use this function and render a list of pages inside.
const Pagination = ({ currentPage, total, limit, onPageChange }) => {
const pagesCount = Math.ceil(total / limit);
const pages = range(1, pagesCount);
return (
<ul className="pagination">
{pages.map((page) => (
<PaginationItem
page={page}
key={page}
currentPage={currentPage}
onPageChange={onPageChange}
/>
))}
</ul>
);
};
As you can see here we created an array of pages
and rendered additional component PaginationItem
for every single page.
Now let's add this PaginationItem
component. But here we need to install additional library to create classes easier and it is called classNames
.
npm install classnames
const PaginationItem = ({ page, currentPage, onPageChange }) => {
const liClasses = classNames({
"page-item": true,
active: page === currentPage,
});
return (
<li className={liClasses} onClick={() => onPageChange(page)}>
<span className="page-link">{page}</span>
</li>
);
};
Here we added our PaginationItem
component. It's just a li
with click event and a page number. Also here we generated liClasses
with classNames
library. We apply active
class only when the page that we render equals currentPage
.
As you can see in browser our pagination is fully functional and we can change the active page.
It is not so difficult to implement pagination on your own.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done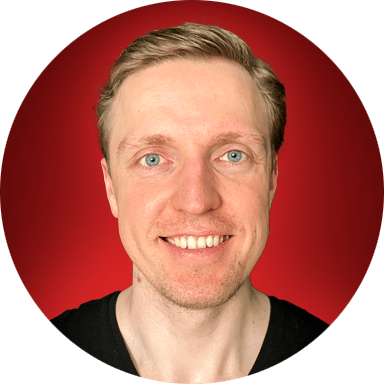