Building an Angular Search Bar with Angular Autocomplete: A Step-by-Step Tutorial
In this post you will learn how to implement a search bar inside Angular.
Project
Here I already prepared several things. First of all we have an API localhost:3004/articles
which returns for us an array of objects with id
, slug
and title
.
I already created an interface for our future article that we want to search.
// src/app/article.interface.ts
export interface ArticleInterface {
id: string;
slug: string;
title: string;
}
This is exactly the format of our article that we get from the backend.
Secondly I prepared ArticleService
which gets articles from the API.
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs';
import { ArticleInterface } from './article.interface';
@Injectable()
export class ArticlesService {
constructor(private http: HttpClient) {}
getArticles(searchValue: string): Observable<ArticleInterface[]> {
return this.http.get<ArticleInterface[]>(
`http://localhost:3004/articles?title_like=${searchValue}`
);
}
}
Here we have a getArticles
method and the most important part is that we must provide a searchValue
inside in order to make API call. As we implement searching by specific string it makes a lot of sense.
Fetching article
The first thing that we need to implement is fetching of the articles inside AppComponent
.
export class AppComponent implements OnInit {
searchValue = ''
articles: ArticleInterface[] = [];
constructor(
private articlesService: ArticlesService,
) {}
ngOnInit(): void {
this.fetchData();
}
fetchData(): void {
this.articlesService.getArticles(this.searchValue).subscribe((articles) => {
this.articles = articles;
});
}
}
Here we injected articlesService
in our AppComponent
and we are fetching data on initialize. Inside fetchData
method we call getArticles
method and provide an empty searchValue
inside.
Creating form for search bar
Now we need to create a form for our search bar. For this we want to use Reactive Forms as the best solution to create forms in Angular.
export class AppComponent implements OnInit {
...
searchForm = this.fb.nonNullable.group({
searchValue: '',
});
constructor(
...
private fb: FormBuilder
) {}
onSearchSubmit(): void {
this.searchValue = this.searchForm.value.searchValue ?? '';
this.fetchData();
}
}
Here we defined our searchForm
with just searchValue
and onSearchSubmit
function. Inside it we assign searchValue
from the form and call fetchData
again.
Preparing HTML
Now we must write markup for our search bar. I already prepared all CSS that we need for search bar so we don't need to spent time writing it.
// src/app/app.component.css
.container {
margin: 0 auto;
width: 500px;
margin-top: 50px;
}
.searchBar {
display: flex;
align-items: center;
justify-content: center;
}
.searchBarClear {
margin-left: 10px;
cursor: pointer;
}
.searchBarInput {
heigth: 30px;
width: 300px;
font-size: 16px;
}
.articlesTable {
margin-top: 80px;
text-align: center;
}
.article {
margin: 10px 0;
}
Now let's write markup for our search bar.
<div class="container">
<form
[formGroup]="searchForm"
class="searchBar"
(ngSubmit)="onSearchSubmit()"
>
<input
type="text"
placeholder="Search..."
formControlName="searchValue"
class="searchBarInput"
/>
</form>
<div class="articlesTable">
<div class="article" *ngFor="let article of articles">
{{ article.title }}
</div>
</div>
</div>
Here we binded a searchForm
to the form that we created and provided an input inside. Also we rendered a list of our articles from the articles
property.
Let's check if it's working.
As you can see we can search for articles on the backend by making an API call every single time when our form is submitted.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done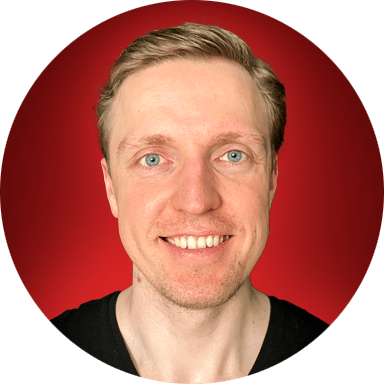