Building React Alert Popup and React Notification Components: Step-by-Step Guide
In this video we will implement together a React alert popup that you can use in your projects without any additional libraries.
And here we need to implement 2 different things. First of all we need an alert component that will just render the information and style it and secondly we need something which will allow us to call this React alert from any place. We will do it with React context.
Creating alert component
First of all let's start to implement our alert component. And we won't have any props inside it because we will get all information later with React context. Must importantly in our application there will be only one place where we render our alerts and this is the top of the screen.
const Alert = () => {
const alert = {
text: 'This is our custom alert',
type: 'success'
}
return <div>{alert.text}</div>;
};
Here we created a test property alert
. We will remove it later. Now let's add some styling.
const alertStyles = {
padding: "16px",
borderRadius: "6px",
fontSize: "16px",
fontWeight: 400,
};
const severityStyles = {
success: {
color: "#0f5132",
background: "#d1e7dd",
},
info: {
color: "#055160",
background: "cff4fc",
},
warning: {
color: "#664d03",
background: "fff3cd",
},
danger: {
color: "#842029",
background: "#f8d7da",
},
};
const Alert = () => {
const alert = {
text: 'This is our custom alert',
type: 'success'
}
const fullStyles = {
...alertStyles,
...severityStyles[alert.type],
};
return <div style={fullStyles}>{alert.text}</div>;
};
Here we created alertStyles
which are always there. We also created severityStyles
which brings us different colors to our component based on provided type
.
Now we just need to render our component in layout.
const App = () => {
return (
<div>
<h1>Hello monsterlessons</h1>
<Alert />
</div>
);
};
Calling alert
Now our component is completely done and we must implement calling of our alert from any place. For this we need to create Alert context.
// src/alert.context.jsx
const AlertContext = createContext();
export const AlertProvider = ({ children }) => {
const [alert, setAlert] = useState(null);
return (
<AlertContext.Provider value={[alert, setAlert]}>
{children}
</AlertContext.Provider>
);
};
export default AlertContext;
Here we created AlertContext
and AlertProvider
. Inside we created a state for alert information and setAlert
which we both make available to outside.
Now we need to wrap our markup with this provider.
// src/main.jsx
import { AlertProvider } from "./alert.context";
ReactDOM.createRoot(document.getElementById("root")).render(
<AlertProvider>
<App />
</AlertProvider>
);
Now we can trigger alert from our App
component. Let's create 2 buttons for this.
const App = () => {
const [, setAlert] = useContext(AlertContext);
const showAlert = (type) => {
setAlert({
text: "This is a custom alert",
type,
});
};
return (
<div>
<h1>Hello monsterlessons</h1>
<Alert />
<button onClick={() => showAlert("danger")}>Show danger</button>
<button onClick={() => showAlert("success")}>Show success</button>
</div>
);
};
Here we used useContext
to get access to our AlertContext
. We also have 2 button which show different alert. The only like that we need to call in order to show our alert is setAlert()
.
Now we can update the logic of our Alert
component to use alert information from our AlertContext
.
const Alert = () => {
const [alert] = useContext(AlertContext);
if (!alert) {
return null;
}
...
return <div style={fullStyles}>{alert.text}</div>;
};
As you can see we render alert only if there is information inside AlertContext
.
Removing alert
The last thing to implement is removing of the alert after period of time as we don't want to see it indefinitely.
export const AlertProvider = ({ children }) => {
...
const timerRef = useRef(null);
useEffect(() => {
if (timerRef.current) {
clearTimeout(timerRef.current);
}
timerRef.current = setTimeout(() => {
setAlert(null);
}, 5000);
}, [alert]);
...
};
Inside our AlertProvider
we added useEffect
which will trigger every time when we change our alert
. After 5 seconds it will be removed.
As we want to restart timeout every time when we change our alert we clear it beforehand.
As you can see in browser our alert is fully implemented and is automatically removed from the page.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done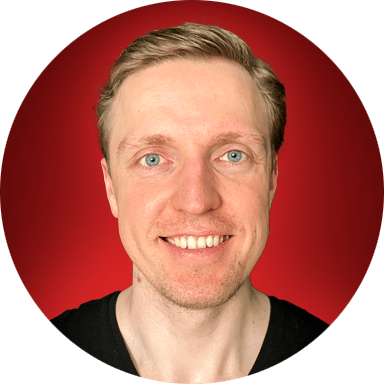