Can ChatGPT write CSS for us?
In this post we will look if ChatGPT can help us to write CSS faster and more efficiency. What I want to build today is a registration form with email, username and password. Also we need 2 links on the bottom for login and resetting password.
This is a typical registration form that we want to implement responsively. Now let's try to get from ChatGPT as much as possible to avoid writing boilerplate.
Step 1
Create pretty HTML form with email username and password in HTML and CSS
Here is an answer of ChatGPT.
<style>
.form-container {
width: 500px;
margin: 0 auto;
border: 1px solid #ccc;
padding: 20px;
box-shadow: 2px 2px 5px rgba(0, 0, 0, 0.1);
}
.form-container h1 {
text-align: center;
margin-bottom: 20px;
}
.form-container input[type="text"],
.form-container input[type="email"],
.form-container input[type="password"] {
width: 100%;
padding: 12px;
border: 1px solid #ccc;
margin-bottom: 10px;
box-sizing: border-box;
}
.form-container input[type="submit"] {
width: 100%;
background-color: #4caf50;
color: white;
padding: 14px;
border: none;
cursor: pointer;
font-size: 14px;
}
.form-container input[type="submit"]:hover {
background-color: #45a049;
}
</style>
<div class="form-container">
<h1>Registration Form</h1>
<form>
<label for="email">Email</label>
<input type="email" id="email" required>
<label for="name">Name</label>
<input type="text" id="name" required>
<label for="password">Password</label>
<input type="password" id="password" required>
<input type="submit" value="Submit">
</form>
</div>
As you can see ChatGPT created for us a nice form-container
with correct markup. What I don't like here is styles for elements based on type and now a specific class.
This is how it look inside browser. As you can see we already got quite a lot, it is already styled and all these fields are looking much better than a default form.
Step 2
The next thing that I want to do is wrap every label and input in additional container. In this case it will be easier to style it.
Wrap label and input in additional div
<div class="form-container">
<h1>Registration Form</h1>
<form>
<div class="form-group">
<label for="email">Email</label>
<input type="email" id="email" required>
</div>
<div class="form-group">
<label for="name">Name</label>
<input type="text" id="name" required>
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" id="password" required>
</div>
<input type="submit" value="Submit">
</form>
</div>
As you can see ChatGPT wrapped every group in the additional element with form-group
class. It even added 10px
margin in CSS so every group is nicely separated.
Step 3
Now I want to get rid of input[type="email"]
and write a normal class here.
add classes for form, inputs, labels and submit button instead of input type
<style>
...
.form-container .form-control {
width: 100%;
padding: 12px;
border: 1px solid #ccc;
box-sizing: border-box;
}
.form-container .btn {
width: 100%;
background-color: #4caf50;
color: white;
padding: 14px;
border: none;
cursor: pointer;
font-size: 14px;
}
.form-container .btn:hover {
background-color: #45a049;
}
</style>
<div class="form-container">
<h1>Registration Form</h1>
<form class="form">
<div class="form-group">
<label class="form-label" for="email">Email</label>
<input class="form-control" type="email" id="email" required>
</div>
<div class="form-group">
<label class="form-label" for="name">Name</label>
<input class="form-control" type="text" id="name" required>
</div>
<div class="form-group">
<label class="form-label" for="password">Password</label>
<input class="form-control" type="password" id="password" required>
</div>
<input class="btn" type="submit" value="Submit">
</form>
</div>
As you can see ChatGPT created styles for form-label
, form-control
, btn
and updated CSS correctly.
Realistically we already got enough markup to start updating CSS on our own without ChatGPT. For now it doesn't make that much difference if we use ChatGPT or not.
Step 4
Now I want to change the font size of our labels and our inputs.
make form-label class 15px and form-control 18px
.form-container .form-label {
font-size: 15px;
}
.form-container .form-control {
...
font-size: 18px;
}
As you can see ChatGPT make correct changes for us.
Step 5
What we are still missing are 2 links on the bottom of our form.
Add login and forgot password links at the end of our form. make them grey and render on the left and right side using flex.
<style>
.form-container .link {
color: gray;
text-align: left;
}
.form-container .link-right {
color: gray;
text-align: right;
}
.form-container .links {
display: flex;
justify-content: space-between;
}
</style>
<html>
<div class="links">
<a class="link" href="#">Login</a>
<a class="link-right" href="#">Forgot password</a>
</div>
</html>
As you can see in browser our code works but the generated CSS is not the best.
.links
class is totally fine but we don't need text-align
at all. We can remove this lines completely and it will still work.
As you can see ChatGPT doesn't generate everything 100% correct but it can write a lot of boilerplate for us.
Step 6
And the last step that we need is to make our form responsive.
<style>
@media (max-width: 600px) {
.form-container {
width: 90%;
}
}
</style>
As you can see we got just a small change which makes our form responsive.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
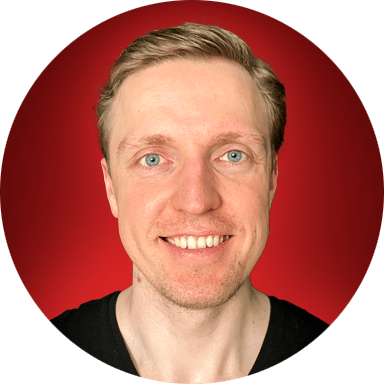