ChatGPT API - Build Own API Using OpenAI API
Every single day I see more and more companies which say that they are "AI Company". Which means company which uses artificial intelligence. But the main point is that they don't have any AI inside their own company. They simply use some API like OpenAI.
This is why in this post I want to show you how to use OpenAI for your own projects so you can also say that you have a startup or a company with artificial intelligence.
So here I registered on the offical website openai.com and we can see some documentation and possibilities to use API of OpenAI. The things that we can use here are: Chat, text completion, embeddings, speech to text, image generation.
Is it free?
The most popular question is obviously "How much does it cost?". This is not a free API. Yes sure you are getting a trial so you can check things out but after that you need to pay.
On the pricing page it says that you only pay for what you use. Which essentially means that it is the same like for example with AWS where you just pay for the requests that you did.
Here you can see that we can use different capabilities and different language models and depending on that you will get different pricing. I can say that it is relatively cheap but you need to check it for your project and budget.
Text completion
Our first step to start using an API is to obtain an API token. For this we must jump to our profile and create a new API key.
Here we must create a new API key that we use later in the application. You must save it somewhere because you can't obtain it again.
// API KEY 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
const app = require("express")();
const bodyParser = require("body-parser");
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.listen(3000, () => {
console.log(`App is started`);
});
Here I already prepared for us a small NodeJS application. I have here Express framework with bodyParser package to parse our body and started server.
Now we must install a package to use openai.
npm install openai
After our package is installed we need to configure openai.
const app = require("express")();
const bodyParser = require("body-parser");
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: "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",
});
const openai = new OpenAIApi(configuration);
Here we created a new Configuration
from openai and provided our API key inside. After this we created openai
instance with this configuration.
The usage of the library has many keys which are not clear without some additional documentation. But on website they have a playground where you can get extended information about every single key.
Here on the right we can configure model, max length and lots of other parameters for our text generation.
Now let's try to implement our API.
app.post("/completion", async (req, res, next) => {
try {
const response = await openai.createCompletion({
model: "text-davinci-001",
prompt: req.body.text,
temperature: 0.4,
max_tokens: 64,
top_p: 1,
frequency_penalty: 0,
presence_penalty: 0,
});
console.log("response", response.data.choices[0]);
res.send(response.data.choices[0].text);
} catch (err) {
next(err);
}
});
Here we created a POST request /completion
where inside we simply make a call to openai
and provide our text from the body in prompt
. The result of our request comes in response.data.choices[0].text
.
As you can see in Postman our request works and OpenAI generated for us a nice Youtube title. This is exactly what all companies nowadays are doing. They simply use OpenAI
inside and nothing more.
Image generation
Now let's try to use image generation. This works in exactly the same way but we use a different function from OpenAI
.
app.post("/imagegeneration", async (req, res, next) => {
try {
const response = await openai.createImage({
prompt: req.body.text,
n: 1,
size: "1024x1024",
});
console.log("response", response.data);
res.send({ url: response.data.data[0].url });
} catch (err) {
next(err);
}
});
Here we called openai.createImage
and provided inside text and the size that we need. Our response with url is in response.data.data[0].url
.
Here we are making our request with the text that we want an image of Happy Potter's universe.
As you can see the image really looks like a well known castle.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done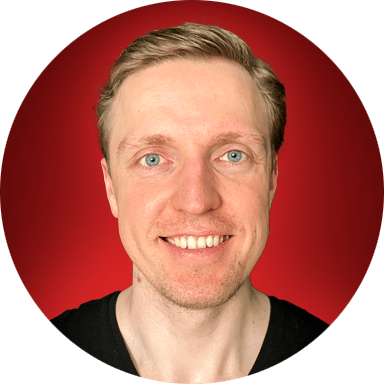