Coding Interview Tasks Javascript - You Must Know Them
In a lot of Javascript interviews you might get a coding exercises. And you must do it directly there in the interview so you don't have any time to google or prepare. This is why in this video I want to show you 5 most popular exercises that I got a lot on the interviews and asked also so you know how to solve them and in what direction you need to think.
Create a function which stores inside a secret word which cannot be changed or accessed from outside
And this is exactly a question to understand if you know closures in JavaScript or not. The main idea is that we must store a function somewhere before we will call it. Only in this case we can store this secret word somewhere before calling.
const someFn() => {
const secret = 'secret'
return () => secret
}
const getSecret = someFn()
console.log(getSecret())
So here we created someFn
which has a secret string inside and it returns a new function. It is important because this is exactly a closure. We will call someFn
and write a result (which is a function) in additional variable. In this case we can use inner function which will return a secret string without allowing to access it directly.
If you get a question regarding closures on the interview you can show this code as it is the easiest example of closure.
How can I clone an object in JavaScript?
To answer this question you must understand that in JavaScript when we assign a object to other variable we simply assigning the reference to this object and we don't clone a value.
let a = {b: 1}
let b = a
b.c = 1
console.log(a, b)
As you can see a and b are exactly the same object because in JavaScript we assign objects and arrays by reference and not by value like primitives.
The solution to this problem is by using spread operator or object assign. And they are working exactly in the same way.
let obj = { a: 1, b: 2};
let clone = { ...obj };
clone.b = 4;
let otherClone = Object.assign({}, obj);
otherClone.b = 6;
clone.b = 4;
// deep cloning
let obj = { a: 1, b: { c: 2 } };
let clone = JSON.parse(JSON.stringify(obj));
clone.b.c = 4;
We created a clone
where we assign new object and spread all properties of obj
inside. The second solution is Object.assign
. Most important part is to provide an empty object as a first parameter.
Count vowels inside a string
This question is typical to check how you can working with transforming arrays or strings, change them or calculate things inside. It doesn't mean that you will get exactly this question but they all are going in the same direction.
const findVowels = str => {
let count = 0
const vowels = ['a', 'e', 'i', 'o', 'u']
for (let char of str.toLowerCase()) {
if vowels.includes(char) {
count++
}
}
return count
}
First of all we must define what are vowels. After that we loop through every symbol of our string and check if the symbol is a vowel. If yes then we increase our counter.
Let's check this out
console.log(findVowels('addgregwo'))
And we get 3 so it is working correctly.
But this code is only good for beginners. Yes we solved the task but our code is far from the advanced level. Instead of for loop we can write a reduce. And if you don't know reduce is a functional way to write loops. And you must try to write as advanced code as possible to show that you are a good developer.
const findVowels = str => {
let count = 0
const vowels = ['a', 'e', 'i', 'o', 'u']
return str.toLowerCase().split('').reduce((acc, char) => vowels.includes(char) ? acc + 1 : acc, 0)
}
So here we used split and reduce to return a counter. Also inside instead of if condition we used ternary operator. If on interview you want to show your skills you must write your code in try and reusable way.
Reverse each word in sentence
The next typical task is to reverse each word in a sentence.
const str = "Welcome to this Javascript Guide!"
console.log(reverseString(str))
Let's try to create this function.
const reverseString(string) => string.split(' ').reverse().join('');
const str = "Welcome to this Javascript Guide!";
console.log(reverseString(str))
So here our function just splits a sentence by words to create an array, then reverses all elements and joins string back.
As you can see we reversed our sentence in a single liner.
Define a function that takes an array of strings and returns the most commonly occurring string.
I saw this task on interview so many times. So you really need to know how to solve it. First I want to show you here a beginner approach and then an advanced approach.
In any solution we want to build an object with such structure.
const obj = {
a: 3,
b: 2,
c: 1
}
In this case the maximum value is what occurs most often.
const mostCommonString = strings => {
const commonStrings = {}
strings.forEach(str => {
if (commonStrings[str] === undefined) {
commonStrings[str] = 1
} else {
commonStrings[str]++
}
})
let maxEntry;
let maxValue = 0;
for (commonString in commonStrings) {
if (commonStrings[commonString] > maxValue) {
maxEntry = commonString
maxValue = commonStrings[commonString]
}
}
return maxEntry
}
So this of all we try to generate object with letters and count as a value. After that we loop through every key of our object and increase maxValue and maxEntry to get the biggest element.
This is the solution and actually the logic is good but we can just change for loops to reduce for more advanced approach.
const mostFrequent = arr => {
const mapping = arr.reduce((acc, el) => {
acc[el] = acc[el] ? acc[el] + 1 : 1
return acc
}, {})
return Object.entries(mapping).reduce((acc, el) => {
return el[1] > acc[1] ? el : acc
}, [null, 0])[0]
}
So here we get the same object with using reduce. After this we use one more reduce to get a maxEntry and maxValue but because we can't use reduce on our object we must use Object.entries first to convert it to array of arrays. Then our object will look like this.
[['a', 3], ['b', 2], ['c': 1]]
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
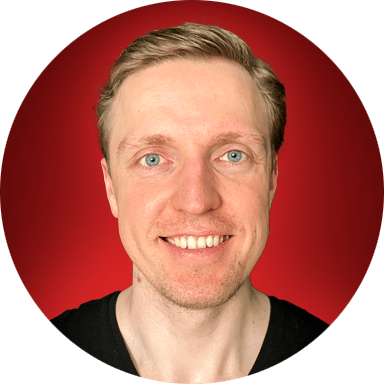