Cookie Consent Popup | Cookie Banner Examples with HTML, CSS, Javascript
In this post we will implement cookie banner just with plain Javascript because you need it for every single website nowadays. This is how it will look like.
We have here a banner on the bottom first time when we enter the page. Inside we have text about agreement to store cookies. When we click accept this banner disappears and after page reload we don't have it anymore.
Why do we need cookie banner?
If you didn't know in Europe every website must show a banner to say that you are using cookies. And actually just for you to know there are different laws in different countries which means actually this banner must be implemented differently. But in most cases it is enough to have just a banner that user can close and won't see anymore after page reload.
But if you really need a banner as a library which supports different countries with their laws then I can highly recommend you to look on cookieconsent. It is not sponsored, I just used it in my own projects. It is written in plain Javascript and you can style it.
But the goal of this video is to write cookie banner by yourself. We will use here plain Javascript, HTML and CSS.
Writing markup
Let's start first with writing markup. Here I have a prepared page which is completely empty.
<!DOCTYPE html>
<html>
<head>
<title>This is the title of the webpage</title>
<link rel="stylesheet" href="main.css" />
</head>
<body>
<script src="main.js"></script>
</body>
</html>
Now let's add markup of our cookie banner.
<div class="cookies-eu-banner">
By clicking ”OK”, you agree to the storing of cookies on your device to
enhance site navigation, analyze site usage, and improve marketing.
<button>Accept</button>
</div>
It is just some text and an Accept button. We also applied cookies-eu-banner
class to style an element.
Now let's write come styles for our banner
.cookies-eu-banner {
background: #444;
color: #fff;
padding: 6px;
font-size: 13px;
text-align: center;
position: fixed;
bottom: 0;
width: 100%;
z-index: 10;
}
.cookies-eu-banner button {
text-decoration: none;
background: #222;
color: #fff;
border: 1px solid #000;
cursor: pointer;
padding: 4px 7px;
margin: 2px 0;
font-size: 13px;
font-weight: 700;
transition: background 0.07s, color 0.07s, border-color 0.07s;
}
.cookies-eu-banner button:hover {
background: #fff;
color: #222;
}
Here we positioned our banner with fixed
at the bottom of the page and added z-index
so it always stays on top. We also styled our Accept button.
Writing Javascript
Our markup is fully ready and we must just write some Javascript logic. And actually inside main.js
I already prepared 2 functions.
const getCookie = (name) => {
const value = " " + document.cookie;
console.log("value", `==${value}==`);
const parts = value.split(" " + name + "=");
return parts.length < 2 ? undefined : parts.pop().split(";").shift();
};
const setCookie = function (name, value, expiryDays, domain, path, secure) {
const exdate = new Date();
exdate.setHours(
exdate.getHours() +
(typeof expiryDays !== "number" ? 365 : expiryDays) * 24
);
document.cookie =
name +
"=" +
value +
";expires=" +
exdate.toUTCString() +
";path=" +
(path || "/") +
(domain ? ";domain=" + domain : "") +
(secure ? ";secure" : "");
};
This 2 functions are not related to our cookie banner. This is getCookie
and setCookie
and typically you will have such utility methods to help you work with cookies.
getCookie
allows us to read just a single cookie by name. setCookie
allows us to update single cookie value and also add additional options if needed.
Actually for us the most interesting part is expiryDays
. Because we want to show this banner again after 30days or 1 year.
In this case cookie will simply disappear and we should not do anything
And actually if you don't want to retype this methods from the screen you can take them from the source code at the end of the post.
Implementing JS logic
Now let's write our code for cookie banner.
(() => {
const $cookiesBanner = document.querySelector(".cookies-eu-banner");
const $cookiesBannerButton = $cookiesBanner.querySelector("button");
$cookiesBannerButton.addEventListener("click", () => {
$cookiesBanner.remove();
});
})();
Here we got our DOM element, it's button and attached a click event to it. Most importantly we wrap our code in IIFE - anonymous function to isolate all our properties and avoid polluting global namespace.
As you can see in browser after clicking Accept our banner disappears.
Now we need to check cookie. But first of all we need to hide our banner at the very beginning. For this let's create new hidden
class.
.hidden {
display: none;
}
<div class="cookies-eu-banner hidden">
...
Now we don't see our banner at the beginning. Let's add cookie checks.
const $cookiesBanner = document.querySelector(".cookies-eu-banner");
const $cookiesBannerButton = $cookiesBanner.querySelector("button");
const cookieName = "cookiesBanner";
const hasCookie = getCookie(cookieName);
if (!hasCookie) {
$cookiesBanner.classList.remove("hidden");
}
$cookiesBannerButton.addEventListener("click", () => {
setCookie(cookieName, "closed");
$cookiesBanner.remove();
});
We get a cookie with name cookiesBanner
. If it is not set then we remove our hidden
class. After clicking on apply we set our cookie with value closed
. In this cases after page reload our banner won't be visible.
As you can see it is not that difficult to implement cookie banner on your own.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done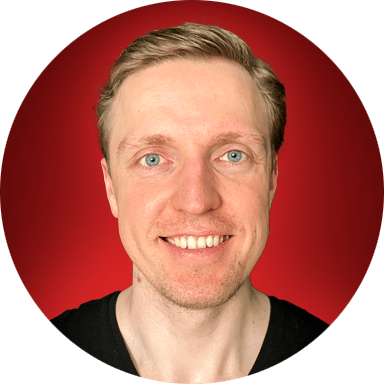