How to Center Elements Using CSS
In this post you will learn all possible ways to center elements in your page just by using plain CSS.
So here I have a completely empty page and this is how my HTML and CSS are looking like.
<!DOCTYPE html>
<html>
<head>
<title>This is the title of the webpage</title>
<link rel="stylesheet" href="main.css"/>
</head>
<body>
</body>
</html>
body {
background: #6886a2;
}
Aligning text
The first possibility that we can use to align elements is text-align:center
. But here is an important point.
We can center only inline elements or inline block elements.
<div class="container">
<div class="content">HELLO CSS</div>
</div>
.container {
text-align: center;
}
.content {
width: 200px;
}
This code won't work because our content
class is a block and we can't center block elements. What we can do to fix it is to set it to inline-block
.
.content {
width: 200px;
display: inline-block;
}
Now it is centered correctly because we treat our div
as an inline block.
And actually we have lots of elements which are inline by default. For example instead of div
we can't write inside an img
.
<div class="container">
<img src="https://picsum.photos/200" />
</div>
.container {
text-align: center;
}
Just with text-align
on the parent our image was centered correctly. Because our image is an inline element.
But it is important to remember that you must attach text-align
to the parent and not to the element itself.
Margin
Another possibility to center our content is margin: 0 auto
.
<div class="container">
<div class="content">HELLO CSS</div>
</div>
.container {
margin: 0 auto;
}
So here we have same 2 blocks and we write margin: 0 auto
on the parent. It is not working because our content
class takes full width.
We can't center element if it takes full width.
First of all we need to put margin on the element that we want to center and not on parent. Secondly we must set width on this element.
.content {
margin: 0 auto;
width: 200px;
}
This code works but a lot of developers don't understand what margin: 0 auto
means at all. It is the same code like this.
.content {
margin-top: 0;
margin-bottom: 0;
margin-left: auto;
margin-right: auto;
width: 200px;
}
It means that we set margins on the left and on the right to auto
and browser positions our element in the center of the page.
If you need to center a block element margin: 0 auto
is one of the easiest ways.
Flex
Both previous options are really old and are supported on all browsers. Nowadays we have flex and grid which allow us to position elements in different ways.
.container {
display: flex;
justify-content: center;
}
So here we set flex
on the parent and used justify-content
to center our child block. It doesn't matter if child element is inline or block it will work in any case.
Using flex is my preferable way to align elements. Why? Because we can align items vertically which we most often want to do.
<div class="container">
<div class="content">HELLO CSS</div>
<img src="https://picsum.photos/200" />
</div>
So here I added an image after our content and it doesn't look good.
We can fix it just with a single rule.
.container {
display: flex;
justify-content: center;
align-items: center;
}
And voila it works like a charm.
This is why from my perspective display:flex
is the most versatile tool.
Grid
The last possibility that we have is to use grid. But actually grid is a bad idea to center elements. With grid you typically want to define of your columns and rows. But we can do something similar to see how it works.
<div class="container">
<div class="content">HELLO CSS</div>
<img src="https://picsum.photos/200" />
</div>
.container {
display: grid;
grid-template-columns: 50% 50%;
align-items: center;
}
Here we have exactly the same markup but in css we used display: grid
. Instead of centering our elements we must set the width of each column in 50% for example. Also we can use align-items
to center them vertically.
As you can see here we get our grid with 2 columns. Also it was positioned vertically.
What to use?
- So nowadays I will use flex to position elements or align them vertically.
- I will use text-align if I simply need to align some text.
- I almost never use
margin: 0 auto
- I use grid only when I really want to get a grid with columns and rows but not for the positioning of the elements.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
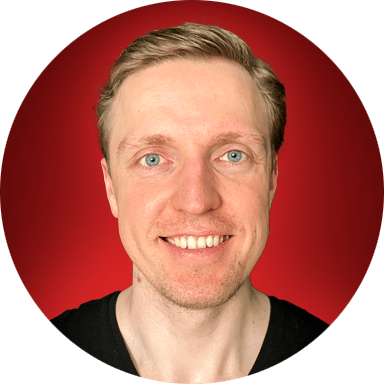