How to Upload Files to Website Using Javascript
In this post you will learn how to implement uploading on your website using Javascript.
It is difficult
I really think that implementing uploading of the files on your website is a difficult task. Why?
First of all we must implement something on the front end - at least a modal or some upload button and style it nicely. Also you might want to implement drag n drop to make it nice and confortable to the user.
Secondly we must implement backend part which will save all our files into the disk, implement API to provide this files from frontend and write information about this files to database.
At some point we might want to move all our files to CDN to make it easier for our server and to deliver files faster.
Also additionally you might want to implement different providers to upload files like Instagram, Dropbox or Facebook.
As you can see there are lots of steps and if you don't have enought knowledge how to do all them correctly it is not an easy task.
Other solution?
This is why there is an easier solution. We can use an additional service which is called Filestack. It is not a free service but it has a free tier so it may be sufficient for small projects.
The main idea of this service is that you simply write several lines of code and you can upload your files directly there.
Now on frontend we simply need to call this modal, we get different providers like Facebook, Instagram, Dropbox, Google Drive our of the box, we don't need to do anything with backend and all files are stored directly in CDN of Filestack.
Additionally during upload we can crop, rotate or make a circle of the photo.
Javascript implementation
First of all I want to show you how to implement Filestack with plain Javascript.
<!DOCTYPE html>
<html>
<head> </head>
<body>
<div>
<button class="upload">Upload file</button>
</div>
<div>
<img width="200" height="200" class="logo" />
</div>
<script src="//static.filestackapi.com/filestack-js/3.x.x/filestack.min.js"></script>
<script src="main.js"></script>
</body>
</html>
Here we have a plain html page with injected Filestack script. Also I added an empty img tag where we will render an image later.
Before we will start with implementing Javascript we must obtain an apiKey from Filestack. You simply need to register an account and copy an apiKey from your profile.
Now lt's add our Javascript code in main.js
.
const button = document.querySelector(".upload");
const image = document.querySelector(".logo");
button.addEventListener("click", () => {
const apiKey = "gewgneoiwjgoisjdiojgiosjiog";
const client = filestack.init(apiKey);
const options = {
onUploadDone: (uploadResponse) => {
console.log("onUploadDone", uploadResponse);
image.setAttribute("src", uploadResponse.filesUploaded[0].url);
},
};
client.picker(options).open();
});
Here we added a click event to our button. Inside we called filestack.init
which created an instance of Filestack. After this client.picker().open()
will open a modal of Filestack with all logic that it provides.
Inside options we provided an onUploadDone
key which will be triggered after our files are uploaded so we can change our frontend.
So as you can see directly after upload our image was available for us on the client.
Also as you can see the url for the image that we get is already from CDN. Which means it is hosted inside Filestack.
Browsing images
After image upload you can manage and remove images in your admin panel inside your account.
If you lost links to your files you can always find them in your admin panel.
Uploading with a framework
Now let's look how to use Filestack with a framework. Here I generated Angular framework but it works in the same way with React.
First of all we must install Filestack dependency and Angular bindings to Filestack.
npm install filestack-js
npm install @filestack/angular
Now we must register Filestack module.
// app.module.ts
import { FilestackModule } from '@filestack/angular';
@NgModule({
...
imports: [
FilestackModule
],
})
export class AppModule {}
Now we can just use Filestack component.
<ng-picker-overlay
[apikey]="filestackApiKey"
(uploadSuccess)="uploadSuccess($event)"
(uploadError)="uploadError($event)"
>
<button>Open picker</button>
</ng-picker-overlay>
Is it super similar to plain Javascript. We must provide apiKey and success callback. It gives us exactly the same modal as with plain Javascript.
Filestack is a nice service which simplifies uploading files to your project.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
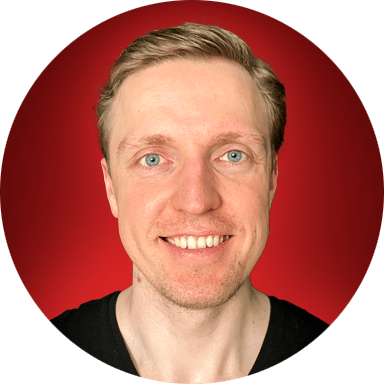