Keyboard Shortcut Keys Using JS - You Should Know This
Today I want to show you how to implement custom keybinds by using Javascript and we will do it on the real project with implementation of undo functionality.
I already prepared here an html with a single input
<form class="form">
<input type="text" placeholder="Add item" class="input" />
</form>
<div class="list"></div>
Here we have a form with our input and a container for a future list of items. So the idea is that we type new element in the input and hit enter and we see our new element in the list.
After this we will implement undo functionality with removing last added element from the list.
Implementing project
First of all let's get all DOM elements inside our Javascript.
const $form = document.querySelector(".form");
const $input = document.querySelector(".input");
const $list = document.querySelector(".list");
I prefixed all of them with $ sign like in jQuery to how that this are DOM nodes.
Now let's add a submit event to our form.
$form.addEventListener("submit", (event) => {
event.preventDefault();
console.log($input.value)
});
It's time to generate a new DOM node and add it to the list.
const items = []
$form.addEventListener("submit", (event) => {
event.preventDefault();
items.push($input.value);
const $newItem = document.createElement("div");
$newItem.innerText = $input.value;
$list.appendChild($newItem);
$input.value = "";
});
So here we did several things:
- We push our new element to items array to implement undo functionality later
- We create a new DOM node with our value and append it to the list
- We clean the value of the input
As you can see our small project is completely ready.
Custom keybinds
Now we are ready for the whole purpose of our project and this is custom keybinds.
To implement custom keybinds we must add keydown event on the document
document.addEventListener("keydown", (event) => {
console.log(event)
});
If we press any key in our page we will get a console log. Which means this is the correct place to get only needed pressed keys and react accordingly.
In our project we want to implement ctrl+z
functionality.
document.addEventListener("keydown", (event) => {
if (event.ctrlKey || (event.metaKey && event.key === "z")) {
console.log("ctrl + z pressed!");
}
});
Inside our event we have all needed information to know what buttons were used. Here we checked for ctrlKey
and metaKey
(for macos) to know if they where pressed. They return us boolean. Also inside event.key
we get the name of the button which we pressed. In our case we are interested only in z
.
As you can see in browser now we get our console.log only if we press ctrl+z
and nothing more. This is exactly how you implement custom hotkeys in Javascript.
Now we just need to add logic of removing items from our list.
document.addEventListener("keydown", (event) => {
if (event.ctrlKey || (event.metaKey && event.key === "z")) {
console.log("ctrl + z pressed!");
if (items.length > 0) {
items.pop();
$list.removeChild($list.lastChild);
}
}
});
If we have any items we use pop
to remove the last one and we remove DOM node after.
Let's check in browser, our code is working.
Hotkeys library
But it is not all. This logic is suitable for small cases but if you have a lot hotkeys in your project, especially in different pages then there is a better solution for you.
I'm talking here about a library hotkeys.js.
What is the point to use a library? If we write lot's of keybinds it is not comfortable to write them every single time like this
if (event.ctrlKey || (event.metaKey && event.key === "z")) {
The idea of this library is simple: there are lot's of predefined hotkeys and we can combine them.
Here is an official example where we combine lots of them
<script src="https://unpkg.com/hotkeys-js/dist/hotkeys.min.js"></script>
<script type="text/javascript">
hotkeys('ctrl+a,ctrl+b,r,f', function (event, handler){
switch (handler.key) {
case 'ctrl+a': alert('you pressed ctrl+a!');
break;
case 'ctrl+b': alert('you pressed ctrl+b!');
break;
case 'r': alert('you pressed r!');
break;
case 'f': alert('you pressed f!');
break;
default: alert(event);
}
});
</script>
So we just call hotkeys and defined needed keybinds inside.
Adding a library
Now let's try to rewrite our code by using a library. First of all we must inject a script.
<script src="https://unpkg.com/hotkeys-js/dist/hotkeys.min.js"></script>
After this we need to just change a single block of code
hotkeys("ctrl+z,command+z", function (event, handler) {
console.log("hotkeys", event, handler);
if (items.length > 0) {
items.pop();
$list.removeChild($list.lastChild);
}
});
This code is similar but much more understandable. Here we call hotkeys
library and write comma separated hotkeys inside.
Also hotkeys
library has lots of other features like different keys, you can register you own hotkeys or define scopes with hotkeys to separate pages or logic in your application.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done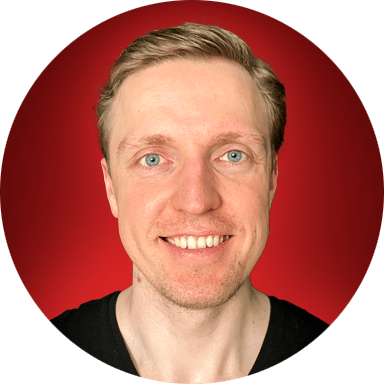